
Web table on browser application contains rows & columns with data on each cell.
Each cell can have text / string, also can have any of other webelements like buttons, checkboxes etc.
A web table has following components –
th – theader – column header
tr – rows
td – cells, consists of actual data
tbody – table with out header
[Refer to the above image – image 1]
If the theader is present & fetching data by identifying the table element, then be careful to exclude the 1st row while fetching data.
Else, you can directly identify the tbody [or table/tbody] & you will get exact no. of rows that has data [excludes the theader].
Scenario –
We will be using demo site – https://qavbox.github.io/demo/webtable/
Check the checkbox from the row, that has cell data = TFS
Let’s see the implementation:
File - test_webTable.py Author - qavalidation.com / youtube.com/qavbox import time from selenium import webdriver from selenium.webdriver.support.select import Select def test_Sample1(): driver = webdriver.Chrome("/Users/skpatro/sel/chromedriver") # driver.maximize_window() driver.get("https://qavbox.github.io/demo/webtable/") driver.implicitly_wait(30) driver.set_page_load_timeout(50) assert "webtable" in driver.title table = driver.find_element_by_id("table01") rows = table.find_elements_by_tag_name("tr") print("rows - " + str(len(rows))) for i in range(len(rows)): columns = rows[i].find_elements_by_tag_name("td") for j in range(len(columns)): if columns[j].text == "TFS": columns[0].click() # print(columns[j].text) time.sleep(5) driver.quit()
Explanation –
To get the no. of rows –
table = driver.find_element_by_id("table01") rows = table.find_elements_by_tag_name("tr") print("rows - " + str(len(rows)))
Identified the table, then the tr tag [rows]
Now the len(rows) provides the row count – 4 [including theader and tbody]
To select a checkbox from the row that has the cell value = “TFS”
table = driver.find_element_by_id("table01") rows = table.find_elements_by_tag_name("tr") for i in range(len(rows)): columns = rows[i].find_elements_by_tag_name("td") for j in range(len(columns)): if columns[j].text == "TFS": columns[0].click()
First for loop will loop over no. of rows.
Then we identified td or cells from each rows
If a column cell has value = TFS, then click checkbox i.e columns[0]
Note –
- If you want to use
contains condition
, then you can useif columns[j].text in "TFS"
- checkbox is present on the 1st column i.e – index 0
Another scenario
use of xpath: if we inspect webtable cells with any inspection tools like selectorshub on webpage, the xpath mostly remains same except the index of tr and td tag…
Let’s see how we can inspect the cell value = TFS
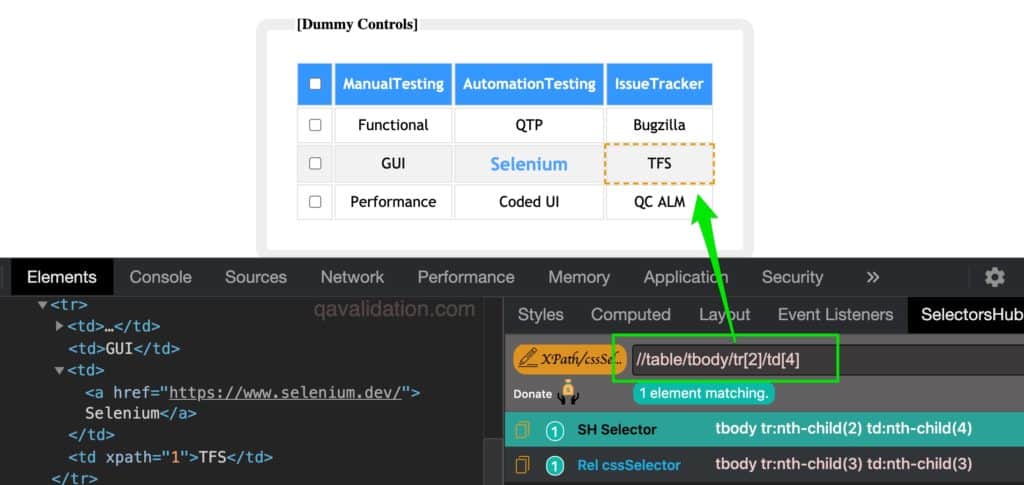
As you can see in above screenshot, item TFS
is on 2nd row and 4th column… [from tbody]
so we can use 2 for loops to iterate the tr and td tags to get the cell values.
Note – TR or TD index starts from 1
let’s see it in action –
driver.find_element_by_xpath("//table/tbody/tr[2]/td[4]")
from tbody, we moved to 2nd row and 4th column.
Hope this helps!
Hello, I have a problem trying to click on an anchor element within a web table any advice?