In one of our post, we have discussed why to use docker containers to test or build something.
Selenium has a project called docker-selenium, which provides the out of box docker containers with Java and browser installed, makes it easy to setup and run the tests.
Selenium has 2 different types of docker containers –
- Standalone containers
- Hub & node containers
Hub & node docker images are separate, where all the node containers need to register with Hub container.
In this post, we will see how to configure Hub & Node containers and run the selenium tests across multiple containers or browsers, also in parallel.
Docker containers download link –
For Hub docker image – https://hub.docker.com/r/selenium/hub/tags
For chrome node image – https://hub.docker.com/r/selenium/node-chrome
For firefox node image – https://hub.docker.com/r/selenium/node-firefox
Docker command to pull images, register & run selenium server –
Two ways to download the images, using
- Individual docker commands
- Docker compose file (recommended)
Using individual docker commands –
We need to create a docker network grid –
docker network create grid
Note – make sure docker desktop should be installed on the system before running above command.
Run below docker command to download the Hub container and run the selenium server
docker run -d -p 4442-4444:4442-4444 --net grid --name selenium-hub selenium/hub:latest
selenium-hub
is the Hub name which we will use to register with node containers below
Let’s now pull/download the chrome node container and register to above Hub container with below command
docker run -d --net grid -e SE_EVENT_BUS_HOST=selenium-hub \
--shm-size="2g" \
-e SE_EVENT_BUS_PUBLISH_PORT=4442 \
-e SE_EVENT_BUS_SUBSCRIBE_PORT=4443 \
selenium/node-chrome:latest
We registred the Hub with node as SE_EVENT_BUS_HOST=selenium-hub
This above commands are applicable when we want to keep all the containers in same network & same machine, so they will be easy to find the Hub name in the network and register with nodes. but if you want to have Hub and node on different machines, then follow below link –
https://github.com/SeleniumHQ/docker-selenium#using-different-machinesvms
Running the docker commands one by one is tedious when we want to download multiple docker images and sometimes we might not remember the commands.
Using Docker compose file –
So we will use the docker-compose to write the commands in a .yml file and include with the project, so this can be version controlled with the team members.
Here is an example of docker-compose.yml file to download docker containers of Hub and node chrome containers, but the same section can be created for other browsers as well.
Let’s create this file under projectDir/docker directory.
docker-compose-hubNode.yml
# To execute this docker-compose yml file use `docker-compose -f docker-compose-v3.yml up` # Add the `-d` flag at the end for detached execution # To stop the execution, hit Ctrl+C, and then `docker-compose -f docker-compose-v3.yml down` version: "3" services: selenium-hub: image: selenium/hub:4.7.2-20221219 container_name: selenium-hub ports: - "4442:4442" - "4443:4443" - "4444:4444" chrome: image: selenium/node-chrome:4.7.2-20221219 shm_size: 2gb depends_on: - selenium-hub environment: - SE_EVENT_BUS_HOST=selenium-hub - SE_EVENT_BUS_PUBLISH_PORT=4442 - SE_EVENT_BUS_SUBSCRIBE_PORT=4443 - SE_VNC_NO_PASSWORD=1 - SE_NODE_MAX_SESSIONS=3 edge: image: selenium/node-edge:4.7.2-20221219 shm_size: 2gb depends_on: - selenium-hub environment: - SE_EVENT_BUS_HOST=selenium-hub - SE_EVENT_BUS_PUBLISH_PORT=4442 - SE_EVENT_BUS_SUBSCRIBE_PORT=4443 - SE_VNC_NO_PASSWORD=1 firefox: image: selenium/node-firefox:4.7.2-20221219 shm_size: 2gb depends_on: - selenium-hub environment: - SE_EVENT_BUS_HOST=selenium-hub - SE_EVENT_BUS_PUBLISH_PORT=4442 - SE_EVENT_BUS_SUBSCRIBE_PORT=4443 - SE_VNC_NO_PASSWORD=1 - SE_NODE_MAX_SESSIONS=3
Refer – https://github.com/SeleniumHQ/docker-selenium/blob/trunk/docker-compose-v3.yml
depends_on: -selenium-hub means the individual node containers will be run after the hub (selenium-hub) runs successfully.
Hub will be having the port 4444.
SE_VNC_NO_PASSWORD=1, To remove the password when viewing the test run on no vnc browser, else the default password is secret
SE_NODE_MAX_SESSIONS=3, refers to how many max browser instances can run in parallel, in this case we can have max of 3 chrome browsers to run the tests in parallel.
To spin up the docker containers, in command prompt navigate to the projectdir/docker directory and run below command (Make sure docker is running on your machine)
docker-compose -f docker-compose-hubnode.yml up -d
Note – docker-compose command will be available default when you install the docker desktop.
This above command will spin the docker containers and can be observed as running status on docker desktop.
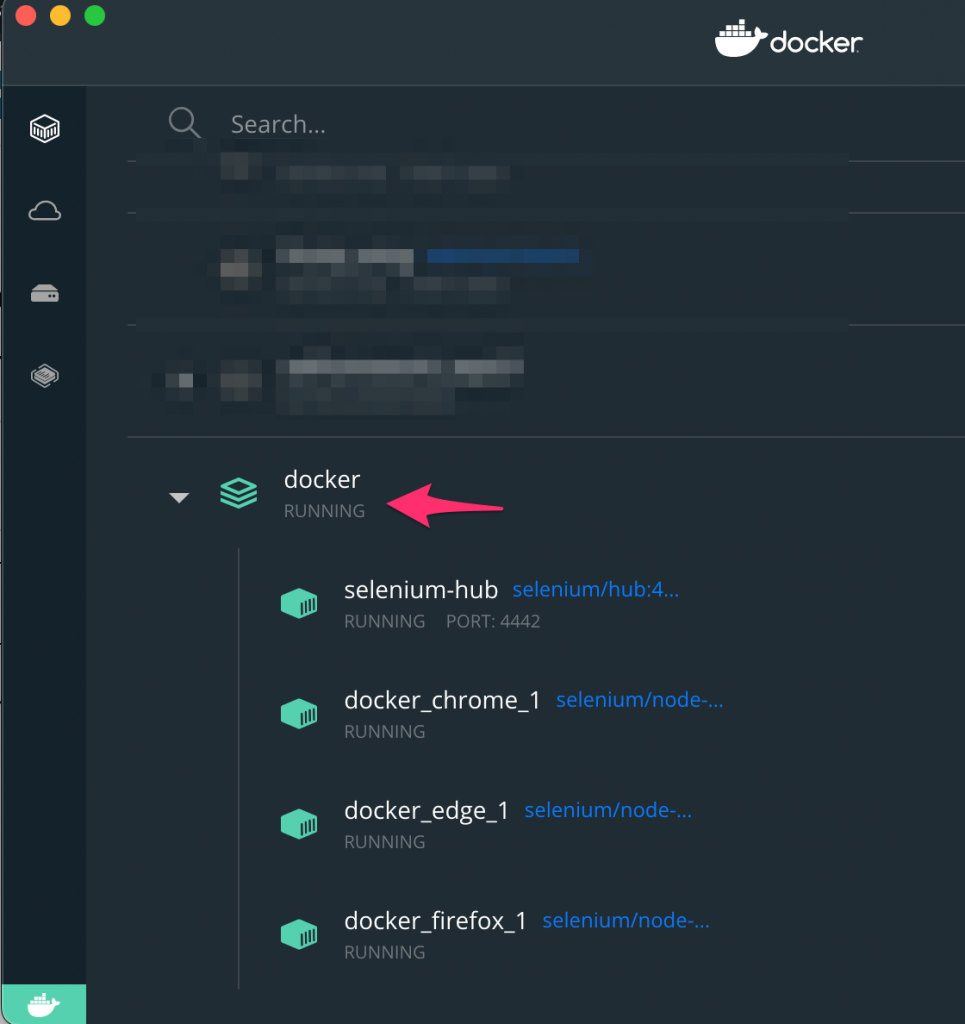
To view the grid dashboard, navigate to this url – http://localhost:4444/ui#
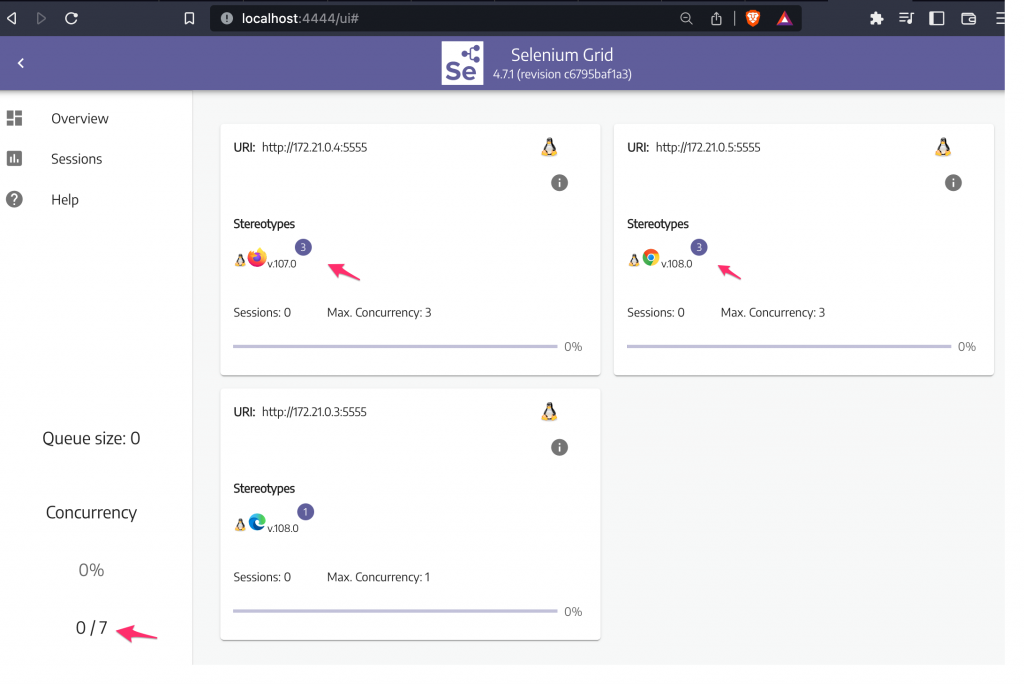
As you can see, we can have 3 chrome, 3 firefox and 1 edge browser (i.e combination of 7 browser instances) to run our tests in parallel. you can play with the SE_NODE_MAX_SESSIONS to alter the no.s.
Run the tests –
Once the docker containers are ready, it’s time to run the tests
We will use our selenium java framework to run the tests, DriverFactory class has all the details to redirect the tests for specific browsers
The driver should redirect to localhost/4444/wd/hub as below –
driver = new RemoteWebDriver(new URL("http://localhost:4444/wd/hub"), co);
To run the test across multiple browsers in parallel, we will use the testng.xml (testng_dockerC_parallel.xml)
Note – You can run the testNG xml file in any ways you prefer, refer Ways to run testng xml file
command to run the testng.xml file with platform = remote (framework will redirect the tests to docker container) –
mvn test -Dplatform=remote -Dsurefire.suiteXmlFiles=testng_dockerC_parallel.xml
observe the execution in url – http://localhost:4444/ui#/sessions
all the 6 tests are running in parallel inside docker containers.
Click on the camera icons for each browser instance to see how the tests running.
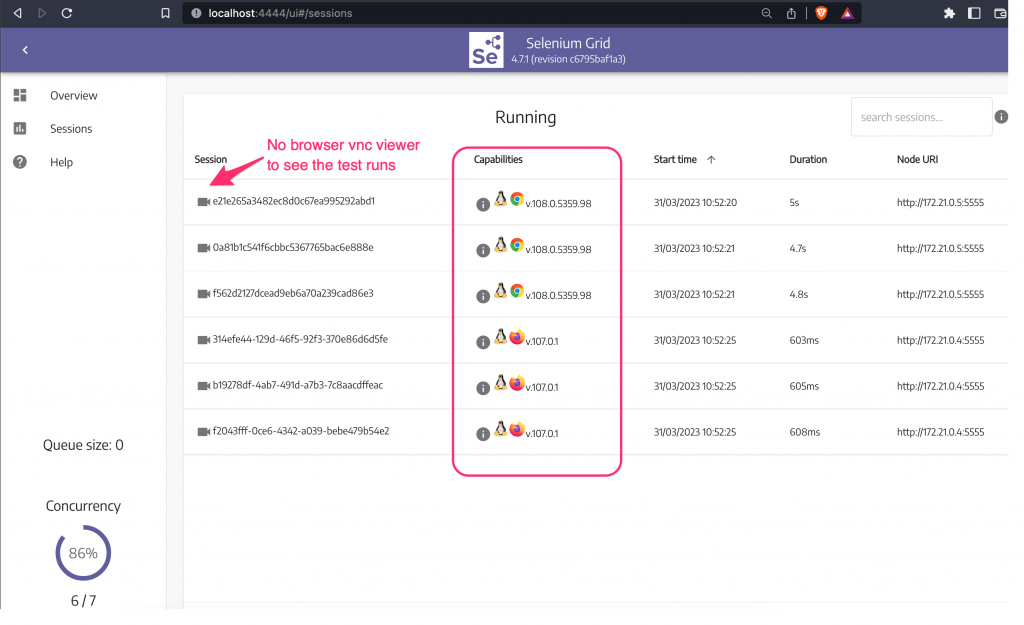
Happy testing!