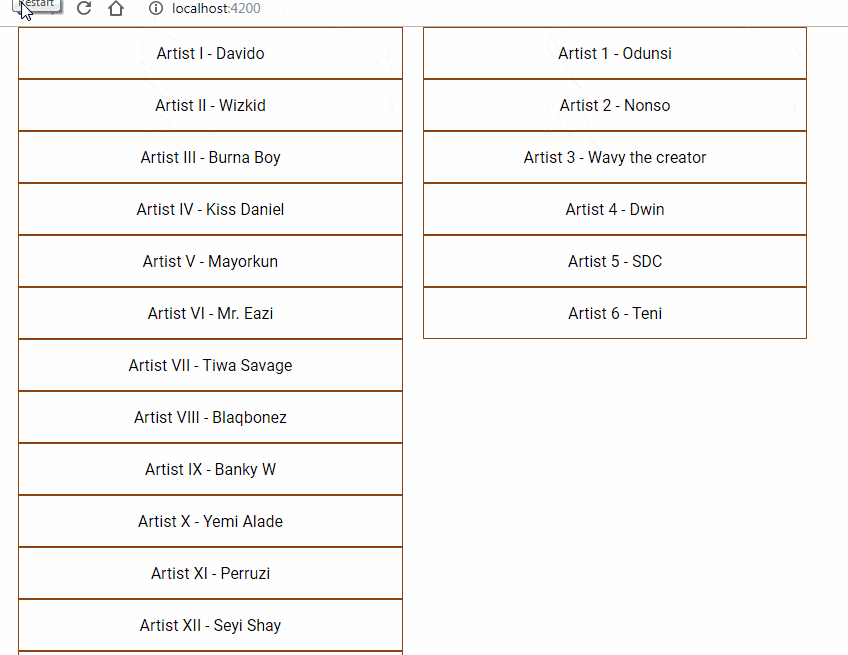
There are several web applications that provides services for drag an element and drop on certain element on browser.
Some scenario usage –
- Can customise menu bar items by drag child elements on parent element.
- Drag and drop files/folders in some hierarchy manner.
- Drag items from left side pane to right side pane to chose preferred options
There are several ways we can automate drag and drop using selenium python library.
Python library provides ActionChains class, that provides several methods to perform sequence of tasks and achieve the drag and drop.
#1
action.drag_and_drop(sourceElement, targetElement)
Drag the source element and drop on top of the target element
#2
action.click_and_hold(source).pause(2).move_to_element(target).perform()
This above will click and hold the source element, then slowly will move to the specified element i.e – on target
Note – when we use sequence of tasks, it’s mandatory to call the perform() method so the individual actions will be run.
#3
action.drag_and_drop_by_offset(source, 170, 10).perform()
If you are not able to identify the target element or you want to drag to specific position on screen, you can use the above method, the parameters are source element, x-offset & y-offset
#4
action.click_and_hold(source).move_to_element_with_offset(target, 100, 100).perform()
This above sequence will drop on target element with a specified x-offset & y-offset, the x & y will be from the top left corner of the target element.
Let’s see the code implementation –
# File - test_dragNdrop.py# Author - qavbox / qavalidation.comimport timefrom selenium import webdriverfrom selenium.webdriver import ActionChainsfrom selenium.webdriver.support.select import Selectdef test_Sample1():driver = webdriver.Chrome("/Users/skpatro/sel/chromedriver")# driver.maximize_window()driver.get("https://qavbox.github.io/demo/dragndrop/")driver.implicitly_wait(30)driver.set_page_load_timeout(50)assert "DragnDrop" in driver.titleaction = ActionChains(driver)source = driver.find_element_by_id("draggable")target = driver.find_element_by_id("droppable")action.drag_and_drop(source, target).perform()# action.click_and_hold(source).pause(2).move_to_element(target).perform()# action.click_and_hold(source).pause(2).move_to_element_with_offset(target, 100, 100).perform()# action.drag_and_drop_by_offset(source, 170, 10).perform()time.sleep(2)print(target.text)assert "Dropped!" in target.textdriver.save_screenshot("/Users/skpatro/sel/dragndrop.png")driver.quit()
Explanation –
After the drop finished, wait for a moment and then you can verify if the element is dropped or not.
The demo site https://qavbox.github.io/demo/dragndrop/
Once the element is dropped [source element on target], the target element text will be changed “Drop here” to “Dropped!”, so we have asserted the text after the chain of actions are performed.
Else, you can take a screenshot to verify the code worked or not.
Hope this helps!