ActionChains
in selenium python provides various ways to automate user interactions on the browser elements like click, mouse move to a particular element, context click, key press etc.
Using ActionChains methods, we can perform one or more sequence of tasks together.
in this post we will see each of the ActionChains exposed methods and learn their usages.
Syntax
action = ActionChains(driver) action.[available methods].perform
ActionChains can be imported from
from selenium.webdriver import ActionChains
Note – You have to call the perform()
at end of each action or task to run.
Click & send_Keys using action
File - test_ActionChainsSample.pyAuthor - youtube.com/qavbox | qavalidation.comimport timefrom selenium import webdriverfrom selenium.webdriver import ActionChainsfrom selenium.webdriver.common.keys import Keysdef test_Sample1():driver = webdriver.Chrome("/Users/skpatro/sel/chromedriver")driver.get("https://qavbox.github.io/demo/signup/")driver.implicitly_wait(30)driver.set_page_load_timeout(50)assert "Registration" in driver.titleaction = ActionChains(driver)username = driver.find_element_by_id("username")action.click(username).perform()time.sleep(3)action.send_keys("QAVBOX").perform()time.sleep(3)action.send_keys_to_element(username, "qavbox").perform()time.sleep(3)driver.quit()
We are working on the demo site – https://qavbox.github.io/demo/signup/
action.click(username).perform()
basically will simply click on the element passed into the method.
There is another method action.click()
, this will click on the position where the mouse is currently focused at.
action.sendKeys(“anyText”) basically sends the character sequence or string into the position where the cursor or mouse is focused at.
action.click(username).perform() action.send_keys("QAVBOX").perform()
2nd line will send the string or enter the string into the username textbox, as the mouse is focused at the username field from 1st line run.
action.send_keys_to_element(element, "sometext")
This above method will send the text directly to the specified element.
Move to an element
username = driver.find_element_by_id("username") action.move_to_element(username).click().send_keys("WATCH_QAVBOX").perform()
We can move mouse to a specified element and perform operations like click on it or expand the sub-child items.
sub-child items – mostly menu items will expand or display the child items when user move the mouse / mouse hover on the menu items [parent items]
Right click – handle context menu items
There are applications which provides some right click options for specific elements like copy, paste, delete, drag etc
These options are basically replaced with the browser specific context or right click menu options
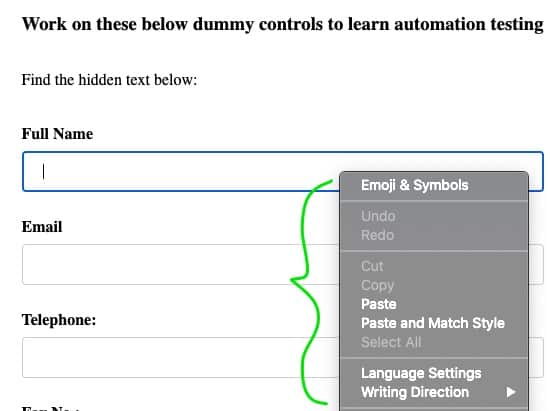
.
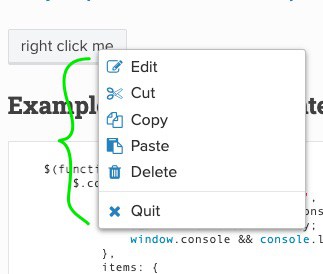
We can’t handle the browser specific options, but using selenium’s context_click()
we can handle the application specific options [as shown on the right above image.]
To handle the context menu option, we will consider this demo site – https://swisnl.github.io/jQuery-contextMenu/demo.html
We will inspect the right click me element and then use the context_click() to expose the right click options and click any of the options
For this we have to perform set of actions or tasks like 1st right click > key press arrow_down > key press arrow-down > key press Enter
Note – ActionChains send_Keys() also helps us to send key press events along with character sequence on the browser screen.
driver.get("https://swisnl.github.io/jQuery-contextMenu/demo.html")time.sleep(3)rightClickEl = driver.find_element_by_xpath("//span[contains(text(), 'right click me')]")action.context_click(rightClickEl).send_keys(Keys.ARROW_DOWN).pause(2)\.send_keys(Keys.ARROW_DOWN).pause(1).send_keys(Keys.ENTER).perform()
1st identify the element, then right click to select a particular context menu option.
The demo site just shows an alert once you click on any menu options, in real time application might perform the actual operation like copy, delete etc, so accordingly you can verify the operation performed or not.
Key press – enter key combinations
In the above example, we have seen how to send individual key press events to an element or browser, but in situations where we might need to send key combinations, example – COMMAND + C [copy in mac os] or CONTROL + C [copy in windows os]
user action for above task would be –
Hold the COMMAND key > press C key > release COMMAND key
ActionChains provides
key_down(key press) to hold a key
key_up(key press ) to release a key
let’s try to automate a scenario on https://qavbox.github.io/demo/signup/
Enter value in username field > copy > then paste in email field
driver.get("https://qavbox.github.io/demo/signup/")action = ActionChains(driver)username = driver.find_element_by_id("username")email = driver.find_element_by_id("email")username.send_keys("QAVBOX")action.key_down(Keys.COMMAND).key_down("A").key_up(Keys.COMMAND).perform()time.sleep(2)action.key_down(Keys.COMMAND).key_down("C").key_up(Keys.COMMAND).perform()time.sleep(2)action.click(email).key_down(Keys.COMMAND).key_down("V").key_up(Keys.COMMAND).perform()time.sleep(2)driver.quit()
on the line username.send_Keys(), we have already focused on the username field, so the next line is performing the COMMAND + A to select all text
then, COMMAND + C to copy
then we are clicking on the email field to have focus and then performed COMMAND + V to paste.
This above examples are for demo purpose to show the capabilities of ActionChains actions
You can explore the same using any other sites.
Hope this helps!