Cypress is an automation tool to automate browser actions and API calls using Javascript & Typescript.
Cypress benefits –
let’s see couple of benefits of using Cypress –
- Out of box support for Typescript
- Has inbuilt assertion methods to validate
- Automatic waiting
- Takes automatic screenshot and videos
- Cross browser testing support
- Can run tests in CI CD environments
Cypress has listed all those above features in it’s own site –
Refer https://docs.cypress.io/guides/overview/why-cypress#Features
Quick Cypress project setup with Typescript –
Open directory in terminal or command prompt & type
mkdir cypress_e2e
cd cypress_e2e
npm init -y //create package.json
npm install cypress
npm I -D typescript
Open the cypress_e2e folder in visual studio code
Open package.json and edit the Scripts section to –
https://docs.cypress.io/guides/tooling/typescript-support#Configure-tsconfig-json
“scripts”: {
“cypress:open”: “cypress open”,
“cypress:run”: “cypress run”
},
As we are inside the Visual studio code, you can open the integrated terminal and type below command to open the Cypress UI to open the tests
npm run cypress:open
You can select any test from the UI and click to run the tests.
Note – watch the video demo to see each step in detail
Above command will create cypress project structure in your project directory to write your tests.
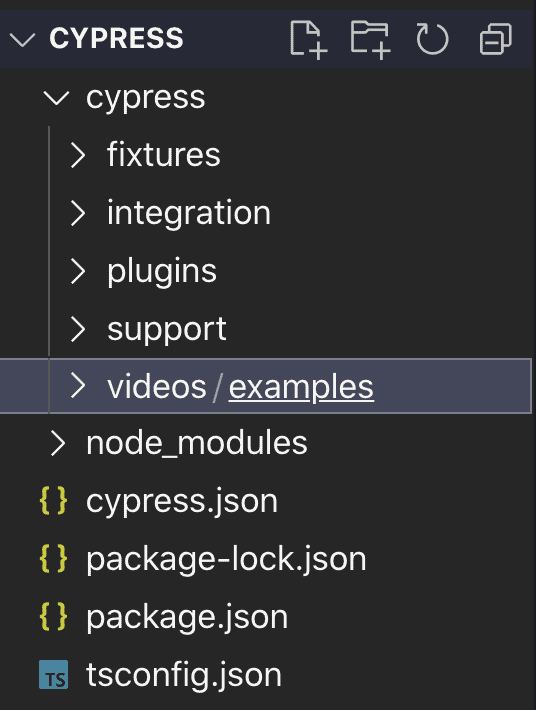
fixtures folder – we can keep global key value data which we can use across our project.
Integration folder is having lots of sample scripts from Cypress to practice and understand the syntaxes.
You can delete them and add your own, even we keep our page objects inside this folder
plugins folder – you can add any 3rd party plugins and use across project
Refer here for list of cypress plugins you can use in project.
support folder – keep all the common methods with respect to your application.
You can write tests in typescript by creating a TS file
for this we need to have tsconfig.json file
tsconfig.json
{ "compilerOptions": { "target": "es5", "lib": ["es5", "dom"], "types": ["cypress"] }, "include": ["**/*.ts"] }
let’s create SampleTS.ts file under integration > sampleTS folder
describe("Reg form", () => { it("enter some value", ()=> { cy.visit("https://qavbox.github.io/demo/signup/"); cy.get("#username").type("qavbox"); cy.get("input[name='home']").click(); }) })
in terminal type & enter – npm run cypress:open
If you navigate to cypress UI, you will see the newly created ts file, click on this file to run tests
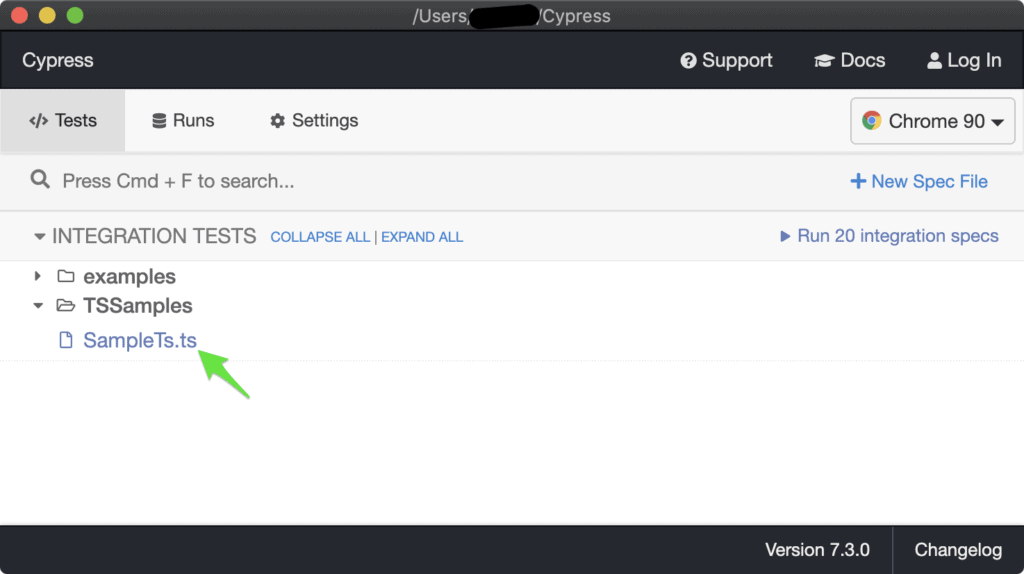
Explanation
cy is a cypress constant, which exposes all of the cypress methods to perform actions.
visit() is to launch the url on browser window
get() uses CSS Selector syntaxes to identify the elements.
type() is to enter text
Stay tuned and subscribe to this video tutorials for more topics!
Hope this helps!