Cypress exposes get() method to identify the browser elements based on the matched selector criteria.
Selector is basically the CSS Selector syntaxes or patterns.
the overloaded methods are –
cy.get(selector) cy.get(selector, options) cy.get(alias) cy.get(alias, options)
Note –
- selector accepts “CSS Selector” patterns
- get() is always chained with cy object
- get() method is used to locate one or more elements
cy.get(selector) example –
//sampleTS.https describe("Reg form", () => { xit("enter some value", ()=> { cy.visit("https://qavbox.github.io/demo/signup/"); cy.get("#username").type("qavbox"); cy.get('input[name=\'home\']').click(); }) })
By default cy.get() waits for 4 sec to load an element, will throw exception if element finding time exceeds 4 sec.
We can chain any other commands with cy.get(), some examples as below
cy.get("#username").type("qavbox");
//to enter some text to text box
cy.get('input[name=\'home\']').click();
//to click an element
If get() returns multiple element matches, then we can validate like below –
cy.get('input[type='text']').should('have.length', '4');
cy.get() returns multiple elements i.e all the textboxes on screen, so we can append other commands like should(‘have.length’, ‘4’) to validate the no. of textboxes should be 4.
or another way we can handle multiple elements like below –
cy.get('input[type='text']').eq(2).type('QAVBOX').should('have.value', 'QAVBOX');
This above code will enter text in to 2nd textbox [if there are multiple textboxes on screen], then verify if the text entered or not.
This above process is called command chaining, cypress evaluates the methods from left to right.
Good! we learned about cy.get(), now let’s see what are the get() options
cy.get(selector, options) example –
There are couple of options Cypress providing to assist locating the element
Options | Description |
log | To print log or not on Cypress UI, default is true |
timeout | Time to wait to identify the element, default is 4000ms |
withinSubject | To find elements with in the specified locator, if null then searches from root |
includeShadowDom | Whether to search inside shadow DOM, configure in cypress.json |
Syntax –
For this demo site – https://qavbox.github.io/demo/delay/
Text appears after 5 sec of clicking Try me button, but default cypress timeout is 4sec, so we can use the timeout option to wait for more time
describe("Reg form", () => { xit("cy get options", ()=> { cy.visit("https://qavbox.github.io/demo/delay/"); cy.get('input[name=\'commit1\']', {log: false}).click(); cy.get('#delay', {timeout: 6000}).should('have.text','I appeared after 5 sec'); }) })
cy.get(‘#delay’ ) will wait for 6sec to locate the element.
log: false with remove the log line from the cypress test runner UI,

below is the screenshot for log: true
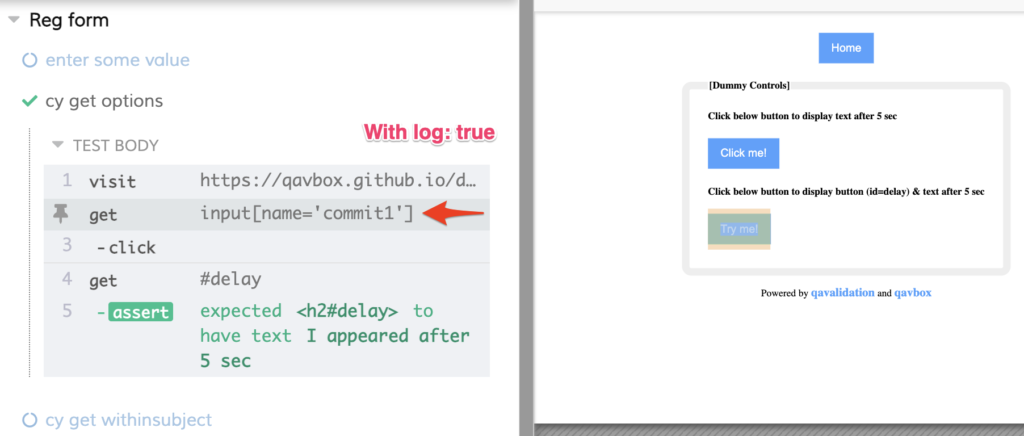
For multiple options with cy.get(), below is the syntax
cy.get('input[name=\'commit1\']', {log: false, timeout: 6000}).click();
withinSubject –
it("cy get withinsubject", ()=> { cy.visit("https://qavbox.github.io/demo/signup/"); cy.get('#username', {withinSubject : document.getElementById('container')}).type("QAVBOX"); })
With in container element, username field will be searched, if matched, then will enter text “QAVBOX”
.find(selector) method
Locating elements based on the matched selector criteria.
One difference between get() & find() is, find() will not be called directly with cy object, rather chained with other cypress methods like
cy.get(selector).find(selector)
.find(selector) .find(selector, options)
options are similar to get() options except the find() don’t have withinSubject option, as find() itself acts as withinSubject.
multiple find() methods can be chained with any other cy commands.
it("cy get find", ()=> { cy.visit("https://qavbox.github.io/demo/webtable/"); cy.get('table').find('thead',{timeout: 6000}).find('th').should('have.length', '4'); })
In the above code, we are identifying the table cells from a table with tagname “table”.
get() method identifies the element as parent and find() will search for matches to the child elements of get(selector).