In this post, we will be executing a typescript file in VS Code
Prerequisites
Nodejs & npm
Refer Install & verify nodejs on windows / Mac OS
TypeScript
To install latest typescript package, Start > Run > cmd, type
npm install -g typescript
to verify, on cmd, type
tsc -v
Visual Studio Code
Download and install from https://code.visualstudio.com/download
Steps to write typescript sample code in vs code
Create a folder TypeScript under C:\ drive.
Open VS code, Select File > Open Folder.. and chose c:\TypeScript (1)
(2) – Create a file
(3) – Create a folder
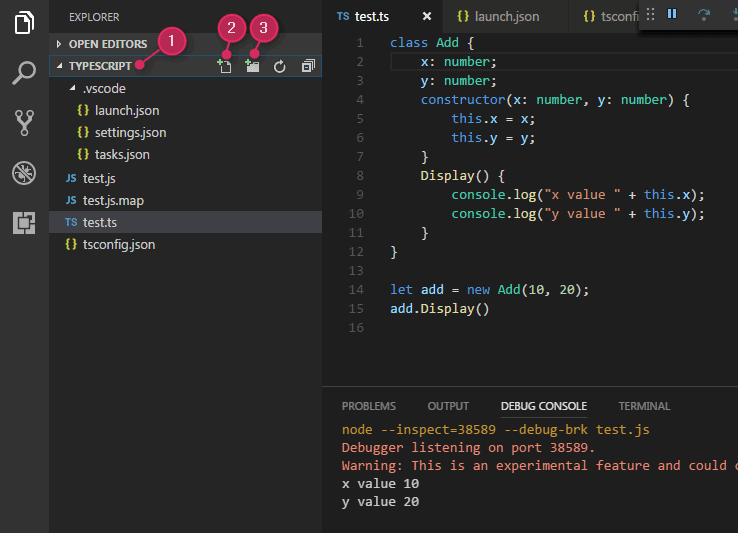
Sample TS code – test.ts
Let’s create a test script file with filename test.ts [under TypeScript folder, as shown above screenshot]
Note – Typescript is superset of java script [with some improvements]
class Add { x: number; y: number; constructor(x: number, y: number) { this.x = x; this.y = y; } Display() { console.log("x value " + this.x); console.log("y value " + this.y); } } let add = new Add(10, 20); add.Display()
x & y are numeric variables, so represented as x : number
constructor() is same as Add() – same name as class name or just constructor
let is a typescript variable declaration type [as we have var in JS but with some improvements]
Refer let for more information.
semicolon at end of code statements are optional
test.ts compiles back to test.js and then executes the code
tsconfig.json
Navigate to the project root folder, in Command prompt [CTRL + `] and type tsc –init
{ "compilerOptions": { "target": "es5", "sourceMap": true, "outDir": "./out", } }
launch.json
To debug the code in VS code, we need launch.json, [under .vscode]
Press F5 to create launch.json and edit the content as below –
{ // Use IntelliSense to learn about possible Node.js debug attributes. // Hover to view descriptions of existing attributes. // For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387 "version": "0.2.0", "configurations": [ { "type": "node", "request": "launch", "name": "TS demo", "protocol": "inspector", "sourceMaps": true, "preLaunchTask": "tsc: build - tsconfig.json", "program": "${workspaceRoot}/test.ts", "outFiles": ["${workspaceRoot}/out/*.js"] }, { "type": "node", "request": "attach", "name": "Launch", "address": "localhost", "port": 5858, "outFiles": [] } ] }
Note – You can even do this build or watch task from
- Go to Manu Bar > Terminal > Run Build Task [Shift + Command + B]
- Click on tsc: build – tsconfig.json or tsc: watch – tsconfig.json [compilation in watch mode]
Any errors, you can view under Terminal
tasks.json
For tsc build task, create a file tasks.json under .vscode folder
{ // See https://go.microsoft.com/fwlink/?LinkId=733558 // for the documentation about the tasks.json format "version": "2.0.0", "tasks": [ { "type": "typescript", "tsconfig": "tsconfig.json", "problemMatcher": ["$tsc"], "group": { "kind": "build", "isDefault": true } } ] }
Now to debug the .ts file, key press F5
If you want to run the code without debugging, then
From Menubar > Run > Run without debugging
or directly key press control+F5 [on Mac OS]
You will see the output under Debug Console [View > Debug Console]
Refer https://code.visualstudio.com/docs/editor/debugging for debugging options.