In our previous post, we have seen how to install protractor and it’s prerequisites.
we will setup some more steps to run protractor tests
Create a new file conf.ts
under C:\Protractor\Test\
import {Config, browser} from 'protractor'; export let config: Config = { framework: 'jasmine', capabilities: { browserName: 'chrome', chromeOptions: {'args': ['disable-infobars']} }, specs: [ './specs/calculator.js' ], onPrepare: () => { let globals = require('protractor'); let browser = globals.browser; browser.ignoreSynchronization = true; browser.manage().window().maximize(); browser.manage().timeouts().implicitlyWait(5000); }, seleniumAddress: 'http://localhost:4444/wd/hub', noGlobals: true };
refer configuration library for more options.
Create a spec file calculator.ts
under C:\Protractor\Test\specs
import {browser, element, by, By, $, $, ExpectedConditions} from 'protractor'; import protractor = require('protractor'); describe('Protractor Demo App', function() { var firstNumber = element(by.model('first')); var secondNumber = element(by.model('second')); var goButton = element(by.id('gobutton')); var history = element.all(by.repeater('result in memory')); let value = element(by.xpath("//*[@class='table']/tbody//tr[1]/td[3]")); function add(a, b) { firstNumber.sendKeys(a); secondNumber.sendKeys(b); goButton.click(); } beforeEach(function() { browser.get('http://juliemr.github.io/protractor-demo/'); }); it('should add correctly', function() { add(1, 2); browser.sleep(3000); expect<any>(value.getText()).toEqual('3'); }) it('should have a history', function() { add(3, 4); browser.sleep(3000); add(5, 6); browser.sleep(3000); expect<any>(history.count()).toEqual(2); }) });
Jasmine framework considers each test script as specs,
Describe represents test suite and accepts 2 parameters, description and function name (can pass empty function as well), describe can have any number of test cases ( it blocks)
beforeEach executes every time a it block encounters.
each it block defines one one test case [we should keep each it block independent].
Refer jasmine 2 for more information.
Folder structure should look like
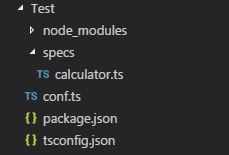
Now key press ctrl + ` to open terminal and enter
webdriver-manager start
You should see a line
INFO - Selenium Server is up and running
Then click on + sign to create another terminal instance and enter
npm test
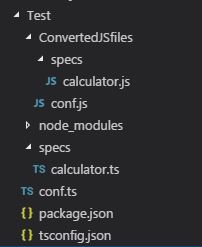
npm test performs following steps- [look for the scripts section of package.json]
tsc – All the *.ts files are transpiled to *.js files and then executes
protractor ConvertedJSfiles/conf.js
Console output –
protractor ConvertedJSfiles/conf.js [19:50:08] I/launcher - Running 1 instances of WebDriver [19:50:08] I/hosted - Using the selenium server at http://localhost:4444/wd/hub Started .. 2 specs, 0 failures Finished in 12.627 seconds [19:50:24] I/launcher - 0 instance(s) of WebDriver still running [19:50:24] I/launcher - chrome #01 passed
2 specs as we have 2 it blocks in calculator.ts file
Complete code can be found under github.com:Protractor-typescript
1 Comment