In this post, we will see how to automate uploading a file using protractor typescript,
Uploading file basically need the file path that needs to be uploaded.
we will identify the upload button which upon click pops up the windows dialog to chose the file, in protractor we can achieve this by using sendkeys() and pass the file path as parameter to the method.
Implementation
We will use a demo site to upload the file
import { browser, element, by, WebElement } from "protractor";let path = require("path");describe("Upload file using protractor", function(){it("Upload file",function(){let filePath = "/Users/myUser/Desktop/testHTML.html";let fpath = path.resolve(__dirname,filePath);browser.ignoreSynchronization = true;browser.get("http://qavalidation.com/demo/");browser.sleep(1000);browser.findElement(by.name("datafile")).then(function(el: WebElement){//scroll till the upload file button om screenbrowser.executeScript("arguments[0].scrollIntoView(true);",el);el.sendKeys(fpath);});browser.sleep(3000);})});
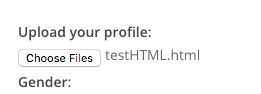
Button “Choose Files” -> el = browser.findElement(by.name("datafile"))
el.sendKeys(fpath)
will upload the file present under fpath, hiding the upload dialog
If you are a beginner to protractor typescript and looking for how to run above code, then follow