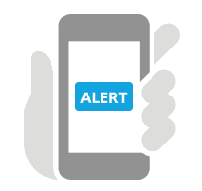
We have seen how to handle alerts for desktop browsers using selenium, the same way we have alerts for mobile or handheld devices.
With handheld devices we have 2 types of alerts
- Native app alerts
- Mobile browser alerts
Let’s understand how each alerts are different and how to handle them using appium
Native app alerts:
Most of the mobile alerts are part of the application but not system alert, and can be identified as usual by using UiAutomatorViewer tool or appium inspector UI (with appium locators).
For iOS apps, you can refer how to inspect iOS apps
For android
we will use sample selendroid app, you can download from here
Observe below screenshot and get the locator details for the “I agree” and “No, no”
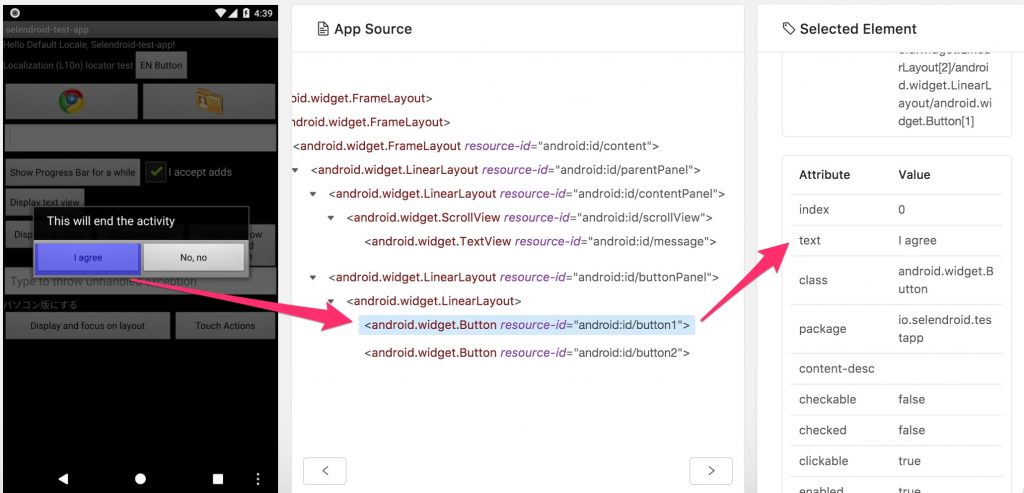
Let’s implement the appium test on the app alert..
public class AlertTest { static DesiredCapabilities cap; static AppiumDriver driver; @BeforeTest static void setup() throws MalformedURLException{ cap = new DesiredCapabilities(); cap.setCapability(CapabilityType.PLATFORM, "Android"); cap.setCapability(CapabilityType.VERSION, "8.1.0"); cap.setCapability("deviceName", "mygeny510"); cap.setCapability("appPackage", "io.selendroid.testapp"); cap.setCapability("appActivity", ".HomeScreenActivity"); driver = new AndroidDriver(new URL("http://127.0.0.1:4723/wd/hub"), cap); } @Test static void Selendroid() throws InterruptedException{ Thread.sleep(2000); driver.findElement(By.name("EN Button")).click(); //Print the alert message System.out.println(driver.findElementById("android:id/message").getText()); //tap on "I Agree" button driver.findElement(By.name("I agree")).click(); } }
For iOS
We will use sample “UI Catalog” iOS app, refer How to install iOS app on iOS simulators
iOS app alerts also can be identified by appium inspector as we have done in android
sample screenshot here
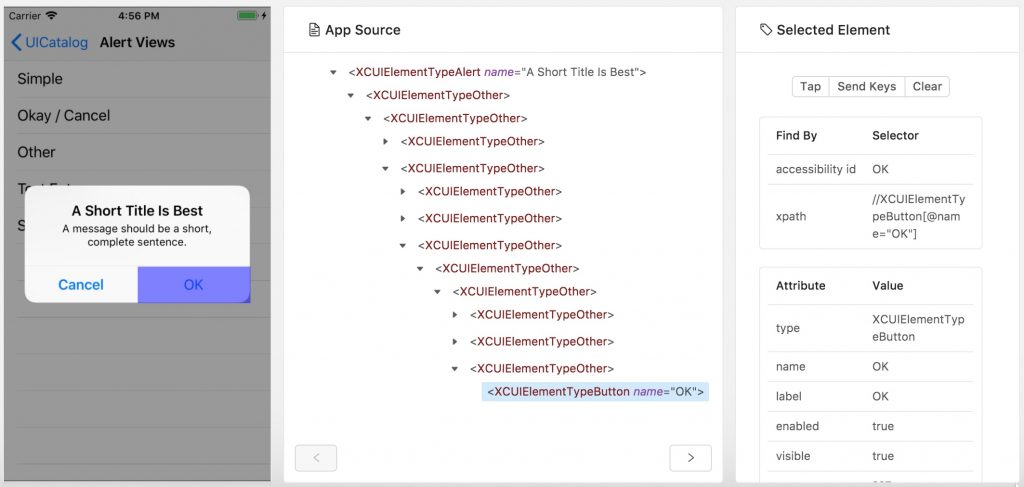
You can use iOS capability and app bundle id as mentioned here and handle the alerts.
Browser alerts:
Alerts from browser actions, like confirmation/warning alerts while
- submitting registration pages
- navigating away from browser while payment is in progress.. etc
These types of alerts can’t be identified by uiautomatorviewer tool, we have to switch to alert and act on them like we did with desktop browser alerts. read handling browser alerts in selenium
driver.get("https://sunilpatro1985.github.io/demo/signup.html"); driver.findElement(By.id("username")).sendKeys("YourName"); WebElement Gender = driver.findElement(By.name("sgender")); Select s = new Select(Gender); s.selectByIndex(2); driver.findElement(By.name("commit")).click(); new WebDriverWait(driver, 30).until(ExpectedConditions.alertIsPresent()); driver.switchTo().alert().accept(); System.out.println("done");
How to handle pop-up windows of the native app. e.g. in the selendroid app there is a button of display popup window. Tapping on which a popup appears. So how to handle that particular popup? Please help.
Did u try identifying the popup, most of the time u should be able to identify as shown in the above screenshot of post.