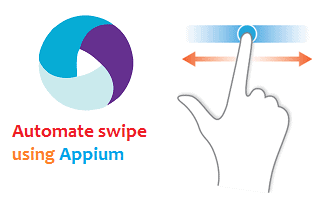
Swipe on mobile handsets can be of from
- Left to right or vice versa
- Top to bottom or vice versa
Let’s see how we can automate swipe in appium.
Implementation
Depending on appium version you are using, implementation defers
On java-client 6.0 onwards, we have use PointOptions.point() to assign coordinates and WaitOptions.waitOptions() to assign duration
new TouchAction((PerformsTouchActions) driver) .press(PointOption.point(startx, Anchor)) .waitAction(WaitOptions.waitOptions(Duration.ofMillis(1000))) .moveTo(PointOption.point(endx, Anchor)) .release().perform();
For Appium Java-Client version 5.0.0 onwards, Swipe() deprecated, alternate solution is to use class TouchAction
new TouchAction().press(int startx, int starty) .waitAction(Duration.ofSeconds(2)) .moveTo(int endx, int endy) .release().perform();
For Appium java-client version less than 5.0.0, use swipe() method
driver.swipe(int startX, int startY, int endX, int endY, int duration);
Start location (x and y axis location) to end location (x and y axis location) and the duration of swipe action.
E.g – If want to move from left to right, change the startx and starty values, but keep constant for startY and endY values.
We will be using Phone application, where we will be swiping from right to left to move Favorites to Call history.
NOTE: Always better to get the width and height of the panel on which we want to swipe rather than hard coding the x and y axis values, as it gets changes from one device to another.
Watch the details here –
Code
package androidTest; import java.net.MalformedURLException; import java.net.URL; import java.time.Duration; import org.openqa.selenium.By; import org.openqa.selenium.Dimension; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.remote.DesiredCapabilities; import org.testng.Assert; import com.mobile.MobileTest.MobileDriver; import io.appium.java_client.AppiumDriver; import io.appium.java_client.PerformsTouchActions; import io.appium.java_client.TouchAction; import io.appium.java_client.android.AndroidDriver; import io.appium.java_client.touch.WaitOptions; import io.appium.java_client.touch.offset.PointOption; public class SwipeTest { public static AppiumDriver<WebElement> driver; public static DesiredCapabilities cap; public static void main(String[] args) throws MalformedURLException, InterruptedException { cap = new DesiredCapabilities(); cap.setCapability("platformName", "Android"); cap.setCapability("deviceName", "pixelxlo26"); cap.setCapability("appPackage", "com.google.android.dialer"); cap.setCapability("appActivity", ".DialtactsActivity"); driver = new AndroidDriver<WebElement>(new URL("http://127.0.0.1:4723/wd/hub"), cap); Assert.assertNotNull(driver); WebElement Panel = driver.findElement(By.id("lists_pager")); //swipe twice SwipeScreen(Panel, driver); SwipeScreen(Panel, driver); driver.quit(); } public static void SwipeScreen(WebElement el, WebDriver driver) throws InterruptedException { WebElement Panel = el; Dimension dimension = Panel.getSize(); int Anchor = Panel.getSize().getHeight()/2; Double ScreenWidthStart = dimension.getWidth() * 0.8; int scrollStart = ScreenWidthStart.intValue(); Double ScreenWidthEnd = dimension.getWidth() * 0.2; int scrollEnd = ScreenWidthEnd.intValue(); new TouchAction((PerformsTouchActions) driver) .press(PointOption.point(scrollStart, Anchor)) .waitAction(WaitOptions.waitOptions(Duration.ofSeconds(1))) .moveTo(PointOption.point(scrollEnd, Anchor)) .release().perform(); Thread.sleep(3000); } }
For appium java-client version <= 5.0.0, follow below code
int wide = contact.getSize().width; int hgt = contact.getSize().height; int startx = (int) (wide * (0.8)); int endx = (int)(wide *(0.2)); int starty = hgt /2 ; int endy = hgt/2; //To move from Fav to all contacts, we need to swipe from right to left driver.swipe(startx, starty, endx, endy, 1000); //or new TouchAction().press(int startx, int starty) .waitAction(Duration.ofSeconds(2)) .moveTo(int endx, int endy) .release().perform();
Explanation of the above code – refer below screenshot
Starting position – we have taken somewhere half of the way of the panel on which we need to swipe.
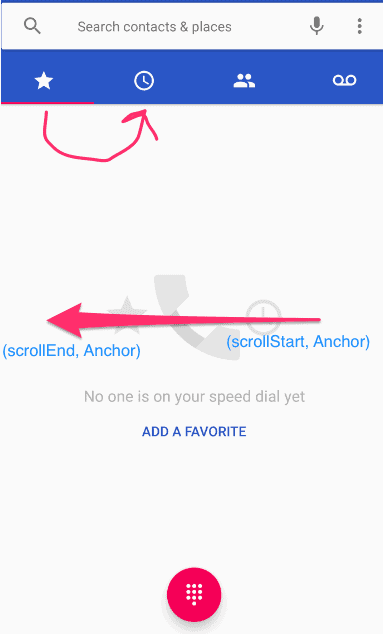
Reference – Appium API documentation
Shortlink – bit.ly/qav-apmswipe
not working
what error u r getting, can I see ur code block and application u r working?
Hi Sunil,
I have a watermark in my application and i want to scroll till that watermark, but when i run the code it says unable to find the element can you please hlep me in this regard
Thanks
Raghvendra
Hi Raghvendra,
Follow bit.ly/qav-apmScroll and retry, or share your app details so I can take a look.
.
Hi,
This code scrolls till the specified time in swipe method, please let me know how to swipe to specific element in the screen (Ex: if there is a element called password in the screen, i want to swipe till password)
HI,
Getting error Original error: Cannot start the ‘com.android.dialer’ application.
Unable to start from Appium also, but able to start from Cmd using “adb shell am start -n com.android.dialer/.DialtactsActivity”
javaclient 6.1.0
java jdk1.8.0_144
Appium server 1.9.1
is you appium server is running before running the tests?
and the appActivity is com.google.android.dialer/.DialtactsActivity
Thank you very much for responding. It’s resolved.
issue was with the activity name. “/” was not required.
not working
which version of appium java-client you are using? as mentioned different version has different approach, provide details of your error…
Appium version 1.18.3
Error Shows in Log: Responding to client with driver. perform touch() result: null
Java does not give any error but touch actions not performed in device
Take an xpath clearly its working for me there is no issue with the code