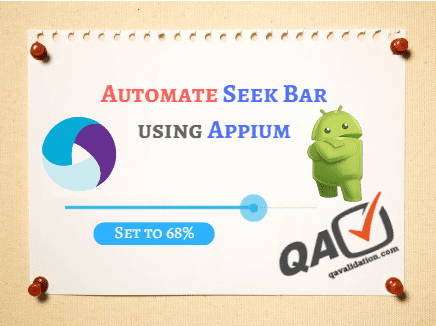
A seek bar in any android handset or emulator is kind of a progress bar but this one is draggable, user can touch on the round shape or thumb, then can move towards right or left.
E.g – increase or decrease of volume or screen brightness.
Let’s see how we can automate the seek bar to move left or right.
Connect your mobile to PC and run below test
Code implementation
[Sample application – ApiDemos.apk]
- Open application
- Tap / click on “Views”
- Scroll till “Seek Bar”
- Drag the seekbar to 70%
DriverFactory.java
package com.mobile.MobileTest; import java.net.MalformedURLException; import java.net.URL; import org.openqa.selenium.WebElement; import org.openqa.selenium.remote.DesiredCapabilities; import org.testng.Assert; import io.appium.java_client.AppiumDriver; import io.appium.java_client.android.AndroidDriver; import io.appium.java_client.ios.IOSDriver; public class DriverFactory { public static AppiumDriver<WebElement> driver; public static DesiredCapabilities cap; public static void Android_LaunchApp() throws MalformedURLException { cap = new DesiredCapabilities(); cap.setCapability("platformName", "Android"); cap.setCapability("deviceName", "pixelxlo26"); cap.setCapability("appPackage", "io.appium.android.apis"); cap.setCapability("appActivity", ".ApiDemos"); driver = new AndroidDriver<WebElement>(new URL("http://127.0.0.1:4723/wd/hub"), cap); Assert.assertNotNull(driver); MobileDriver.setWebDriver(driver); } public static void CloseApp() { driver.quit(); } }
MobileDriver.java
Get and set the driver instance
package com.mobile.MobileTest; import org.openqa.selenium.WebDriver; public class MobileDriver { private static WebDriver Driver; public static WebDriver getDriver() { return Driver; } static void setWebDriver(WebDriver driver) { Driver = driver; } }
TestApp.java
Actual Test to –
Tap on Views
Scroll till Seek Bar
Drag the seek bar
package androidTest; import java.net.MalformedURLException; import org.testng.annotations.*; import com.mobile.MobileTest.*; public class TestApp { @Test public void Test() throws MalformedURLException, InterruptedException { DriverFactory.Android_LaunchApp(); SeekBar seekBar = new SeekBar(); seekBar.clickOnItemViews(); seekBar.scrollTillSeekBar(); seekBar.dragSeekBarTo(70); } @AfterTest public void TearDown() { DriverFactory.CloseApp(); } }
SeekBar.java
package androidTest; import org.openqa.selenium.WebElement; import org.openqa.selenium.support.FindBy; import org.openqa.selenium.support.PageFactory; import com.mobile.MobileTest.MobileDriver; import io.appium.java_client.PerformsTouchActions; import io.appium.java_client.TouchAction; import io.appium.java_client.pagefactory.AppiumFieldDecorator; import io.appium.java_client.touch.offset.PointOption; public class SeekBar { public static TouchAction action; @FindBy(xpath = "//android.widget.TextView[@content-desc='Views']") public WebElement itemViews; @FindBy(xpath = "//android.widget.TextView[@content-desc='Seek Bar']") public WebElement itemSeekBar; @FindBy(className = "android.widget.SeekBar") public WebElement seekBar; public SeekBar() { PageFactory.initElements(new AppiumFieldDecorator(MobileDriver.getDriver()), this); } public void clickOnItemViews() throws InterruptedException { Thread.sleep(2000); itemViews.click(); } public void scrollTillSeekBar() { } public void dragSeekBarTo(int perc) { // get location of seek bar from left int start=seekBar.getLocation().getX(); System.out.println("Startpoint - " + start); //get location of seekbar from top int y=seekBar.getLocation().getY(); System.out.println("Yaxis - "+ y); //Get total width of seekbar int end=start + seekBar.getSize().getWidth(); System.out.println("End point - "+ end); action = new TouchAction((PerformsTouchActions) MobileDriver.getDriver()); //move slider to 70% of width int moveTo=(int)(end * ((float)perc/100)); action.longPress(PointOption.point(start,y)) .moveTo(PointOption.point(moveTo,y)) .release().perform(); } }
Write logic to scroll till SeekBar item in method scrollTillSeekbar(), to get more details, refer scrolling in appium
Run below testng.xml to perform the seekbar operation
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" > <suite name="Suite1" verbose="1" > <test name="TestApps"> <classes> <class name="androidTest.TestApp" /> </classes> </test> </suite>
Hope that helps!
I tried executing the above code. But I am running into this issue:
org.openqa.selenium.WebDriverException: Method has not yet been implemented (WARNING: The server did not provide any stacktrace)
At line : appium.swipe(0,scrollStart,scrollEnd,0,2000);
I am using saucelabs to run my mobile tests
Driver info: io.appium.java_client.ios.IOSDriver
appiumVer “1.5.3”
Please let me know what the issue is
Hi Payal,
Can you update your java-client jar version and retry, I am using java-client version 4.0 or above.
I used the same code for my app, when i run the code i get the below issue
:org.openqa.selenium.WebDriverException: Support for this gesture is not yet implemented. Please contact an Appium dev (WARNING: The server did not provide any stacktrace information)
Command duration or timeout: 11 milliseconds
Build info: version: ‘unknown’, revision: ‘1969d75’, time: ‘2016-10-18 09:43:45 -0700’
System info: host: ‘Rinsofts-Mac-mini-2.local’, ip: ‘192.168.1.120’, os.name: ‘Mac OS X’, os.arch: ‘x86_64’, os.version: ‘10.11.6’, java.version: ‘1.8.0_111’
Driver info: io.appium.java_client.ios.IOSDriver
Java -client version is 5.0BETA.
Hi Vinoth,
From java-client.jar 5.0.0 on wards, swipe() deprecated, so you can replace line 49 with this
new TouchAction(driver).press(0, scrollStart).waitAction(2000).moveTo(0, scrollEnd).release().perform();
Hi,
Some improvement I worked hard on finding out whats not working (i am new in appium):
old line 37: //move slider to 70% of width
int moveTo=(int)(end*0.7); // This will move to 70% of screen0 -> end Seekbar
new:
int moveTo=(int)(widthOfSeekbar*0.7+start); // This will move to real 70% from Seekbar
Best regards
Michi
I have completely updated the post with version of appium java-client 6.0