There are situation when you don’t get exact result after running your test or getting some unknown error or exception and not much details are there on out put, in that situation you might want to observe the line by line execution of code that you have written,
If you run the code in normal way, you can’t see how each and every line executes, so every good editors has a feature called debug
, in which you can navigate through each line of code and verify the variable flow or loopings or the modules that you have written.
Debug navigation shortcut keys
F5 – Step into – Steps into the call – goes to the call definition
F6 – Step over – steps over the call – line by line execution
F7 – Step return – steps out to the caller
F8 – resume – resumes the rest of the execution
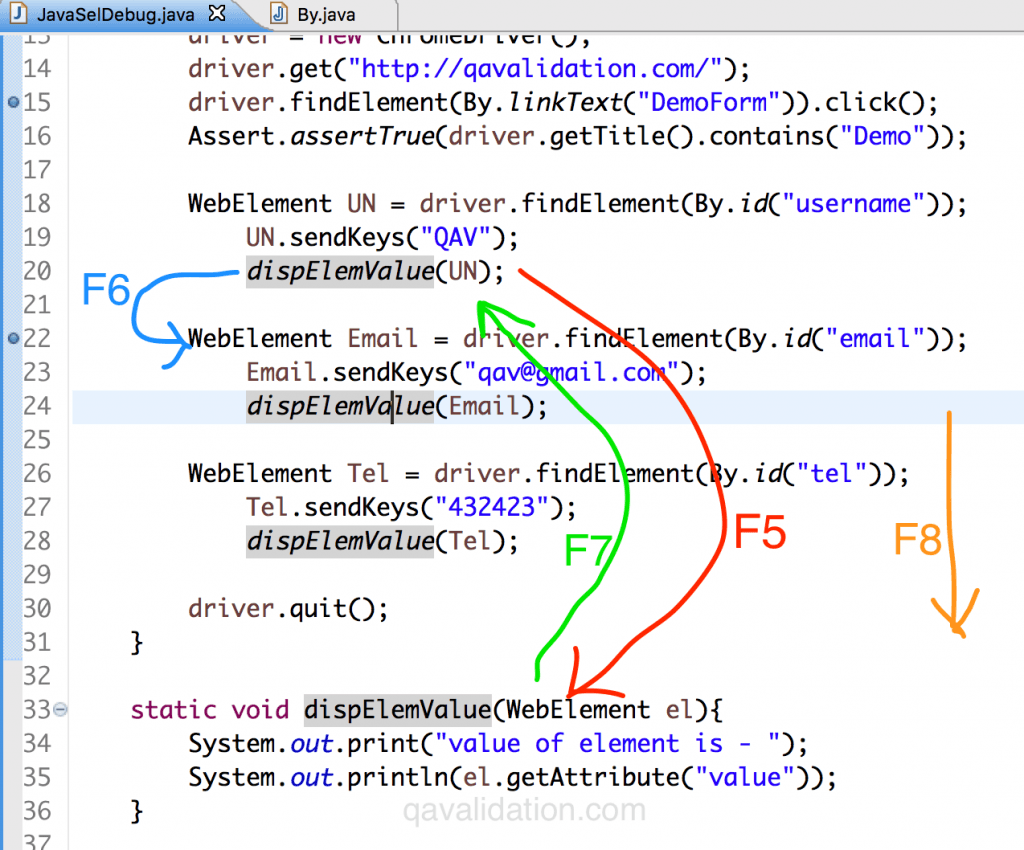
Watch the demo
Here is a video that explains different options you can perform while in debug mode, do watch for more details
Sample code to practice debug –
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.Assert; public class JavaSelDebug { public static void main(String[] args) { WebDriver driver; System.setProperty("webdriver.chrome.driver", "/Users/sunilkumarpatro/sel/chromedriver"); driver = new ChromeDriver(); driver.get("http://qavalidation.com/"); driver.findElement(By.linkText("DemoForm")).click(); Assert.assertTrue(driver.getTitle().contains("Demo")); WebElement UN = driver.findElement(By.id("username")); UN.sendKeys("QAV"); dispElemValue(UN); WebElement Email = driver.findElement(By.id("email")); Email.sendKeys("qav@gmail.com"); dispElemValue(Email); WebElement Tel = driver.findElement(By.id("tel")); Tel.sendKeys("432423"); dispElemValue(Tel); driver.quit(); } static void dispElemValue(WebElement el){ System.out.print("value of element is - "); System.out.println(el.getAttribute("value")); } }