In one of our post, we have seen how to take a screenshot of a browser screen, but unfortunately this logic can not capture the alerts as alerts are not related to browser, so we need to have different approach which we will see in this post.
For alerts, we will use this demo link – alerts
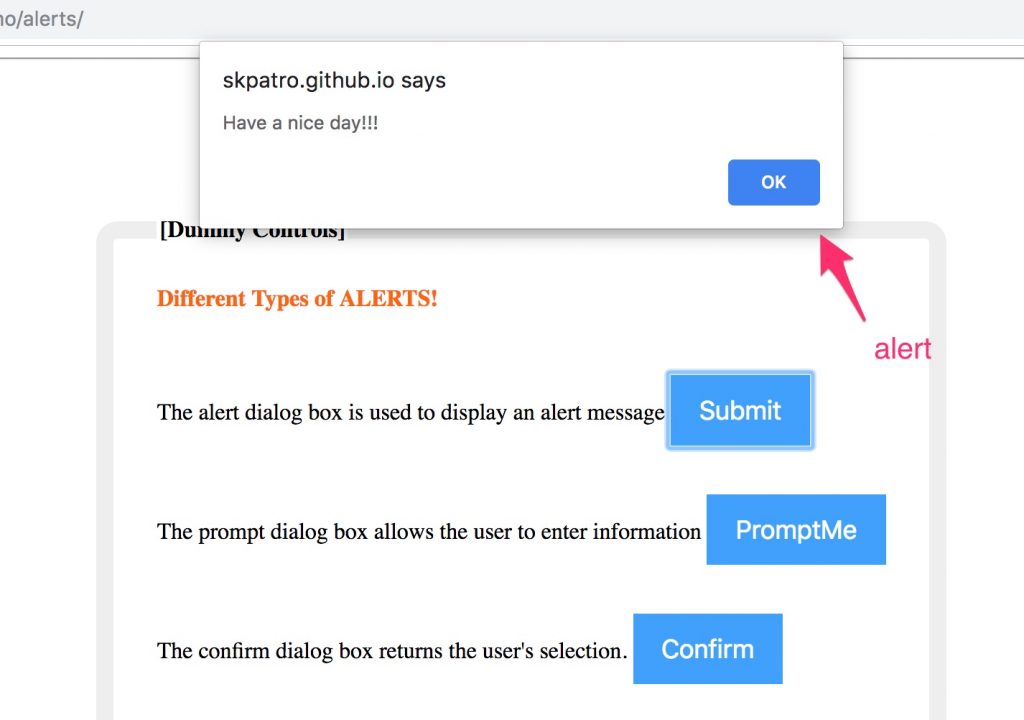
Refer handle alerts in selenium
As we have discussed, TakesScreenshot class can take screenshots of only browser screens, so we will use Robot class which is inbuilt inva so no need to add any extra libraries to project.
Let’s see the implementation
package com.test.MvenTest; import java.awt.AWTException; import java.awt.HeadlessException; import java.awt.Rectangle; import java.awt.Robot; import java.awt.Toolkit; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; import org.openqa.selenium.Alert; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; public class AlertCapture { static WebDriver driver; static WebDriverWait wait; public static void main(String[] args) throws HeadlessException, AWTException, IOException, InterruptedException { System.setProperty("webdriver.chrome.driver", "/Users/sunilkumarpatro/mobile-e2e/BrowserDriver/chromedriver"); driver = new ChromeDriver(); driver.get("https://qavbox.github.io/demo/alerts/"); driver.manage().deleteAllCookies(); wait = new WebDriverWait(driver, 5); driver.findElement(By.name("commit")).click(); wait.until(ExpectedConditions.alertIsPresent()); Alert alert = driver.switchTo().alert(); Thread.sleep(2000); BufferedImage image = new Robot().createScreenCapture(new Rectangle(Toolkit.getDefaultToolkit().getScreenSize())); ImageIO.write(image, "jpg", new File("./alert.jpg")); alert.accept(); //driver.quit(); } }
Make sure you need to capture screenshot before alert.accept();