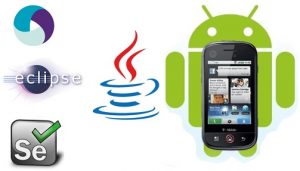
If you are new to appium, please refer below links for the prerequisites
- Eclipse IDE setup
- Appium setup for windows [Mandatory to have the setup before proceeding]
- How to find appium locators
- App Package and activity name
For the appium test: we will be using Selenium, Appium, Eclipse IDE (java) and Android app (.apk) in an emulator or real handset…
Before running appium test on real device or android emulator, make sure we keep the device connected to computer, use adb command to verify.
Install APK file –
Ways to install apps (.apk) on mobile or emulators:
- Install as usual from android play store.
- Using command line
Have the required .apk file on computer, and connect mobile to computer in debug mode.
open command prompt, type
adb install [apk file path]
Download apk file
For appium test, we will use selendroid sample application… [download selendroid-test-app.apk ‘click on view raw to download’]
Appium maven dependency
Get the Appium maven dependency [java-client] from here
Code implementation
import java.util.List; import java.net.MalformedURLException; import java.net.URL; import io.appium.java_client.AppiumDriver; import io.appium.java_client.android.AndroidDriver; import io.appium.java_client.android.AndroidKeyCode; import org.openqa.selenium.By; import org.openqa.selenium.WebElement; import org.openqa.selenium.remote.CapabilityType; import org.openqa.selenium.remote.DesiredCapabilities; import org.testng.Assert; import org.testng.annotations.BeforeTest; import org.testng.annotations.Test; public class SelendroidTest { static DesiredCapabilities cap; static AppiumDriver driver; @BeforeTest static void setup() throws MalformedURLException{ cap = new DesiredCapabilities(); cap.setCapability(CapabilityType.PLATFORM, "Android"); cap.setCapability(MobileCapabilityType.AUTOMATION_NAME, AutomationName.ANDROID_UIAUTOMATOR2); cap.setCapability("deviceName", "emulator-5554"); cap.setCapability("appPackage", "io.selendroid.testapp"); cap.setCapability("appActivity", ".HomeScreenActivity"); driver = new AndroidDriver(new URL("http://127.0.0.1:4723/wd/hub"), cap); } @Test static void Selendroid() throws InterruptedException{ //click on EN button driver.findElement(By.name("EN Button")).click(); System.out.println(driver.findElementById("android:id/message").getText()); driver.findElement(By.name("No, no")).click(); //Click on display text view button and compare the text displayed driver.findElementByName("Display text view").click(); String text = driver.findElement(By.xpath("//*[@content-desc='visibleTestAreaCD']/android.widget.TextView")).getText(); System.out.println(text); Assert.assertEquals(text, "Text is sometimes displayed"); } }
Let’s understand the above code in details:
Code uses testNG, Java and appium…
@BeforeTest
We have capabilities setup for the mobile handset or emulator like DeviceName, Platform, version, app activity, app package etc…
Refer Appium capabilities for more information
then, we have instantiated AndroidDriver object which accepts 2 arguments:
- URL
- Capability object
Under @Test, whatever the code present is same as selenium test.
2 Comments