TextBox is an element where we can enter/input alphanumeric text.
To identify the textBoxes we need to look for the input tag with type=text, [below screenshot]
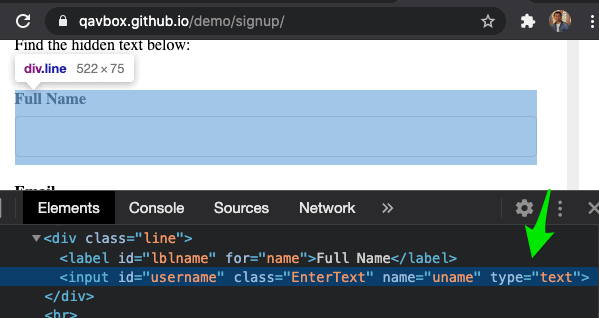
Let’s see some of the actions we can perform with textboxes in selenium:
Enter value to textBox – using sendKeys
method
WebElement txt_FullName = driver.findElement(By.id("username")); txt_FullName.sendKeys("someValue);
After entering text to textBox, we can fetch the entered text using getAttribute("value")
txt_FullName.getAttribute("value");
To clear the text entered, we can use clear() method
txt_FullName.clear();
Let’s see the implementation in detail
package elements; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class TextBoxes { static WebDriver driver; public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/Users/skpatro/sel/chromedriver"); driver = new ChromeDriver(); String UserName = "John K"; driver.get("https://qavbox.github.io/demo/signup/"); WebElement txt_FullName = driver.findElement(By.id("username")); txt_FullName.sendKeys(UserName); //sendKeys method to send text to textBox //Get the entered text using getAttribute("value") System.out.println("UserName Entered: "+txt_FullName.getText()); //OR System.out.println("UserName Entered: "+txt_FullName.getAttribute("value")); //compare if the string entered is correct or not if(UserName.equals(txt_FullName.getAttribute("value"))) System.out.println("TestCase Pass"); //clear the text entered txt_FullName.clear(); } }
Hope this helps!
1 Comment