There are situations where web controls/elements reside inside an inline frame, the inline frame is another document or dom resides inside current HTML document.
iframe can be represented in html as tag name=”iframe”.
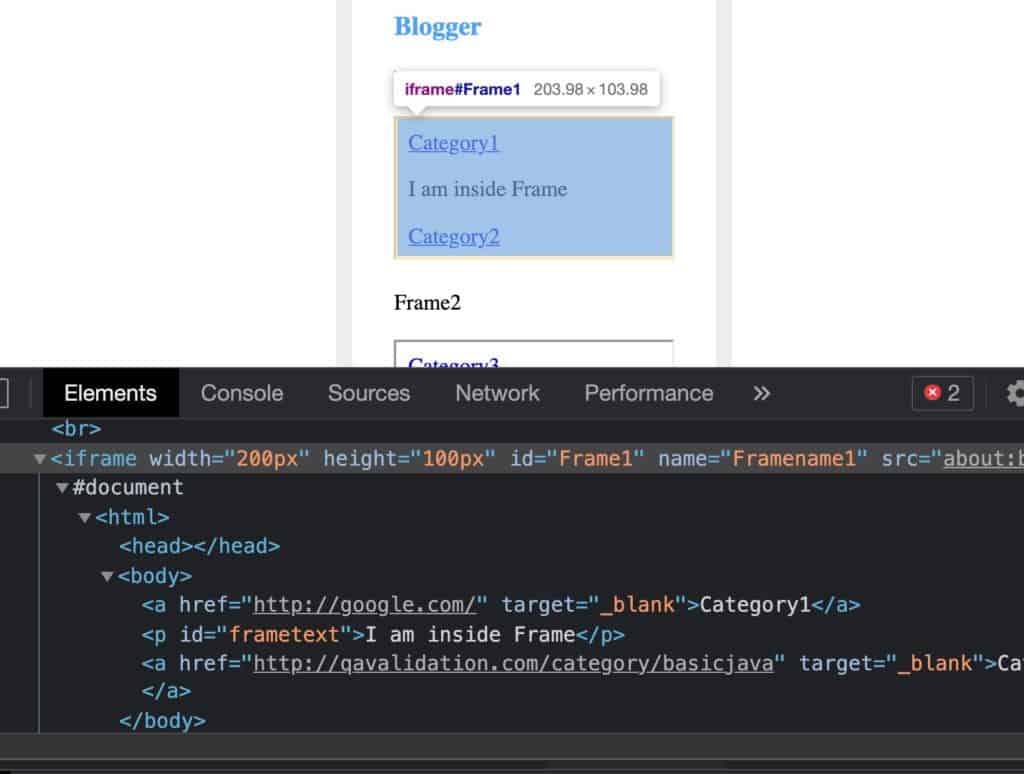
Elements inside an iframe can not be accessed as normal selenium statement – driver.findelement(By...)
need to use,
driver.switchTo().frame(...);
In selenium, how we can identify if web element[s] is/are inside an <iframe …=””> </iframe> ???
Right click on an web element, check if the context menu has any one of the following options –
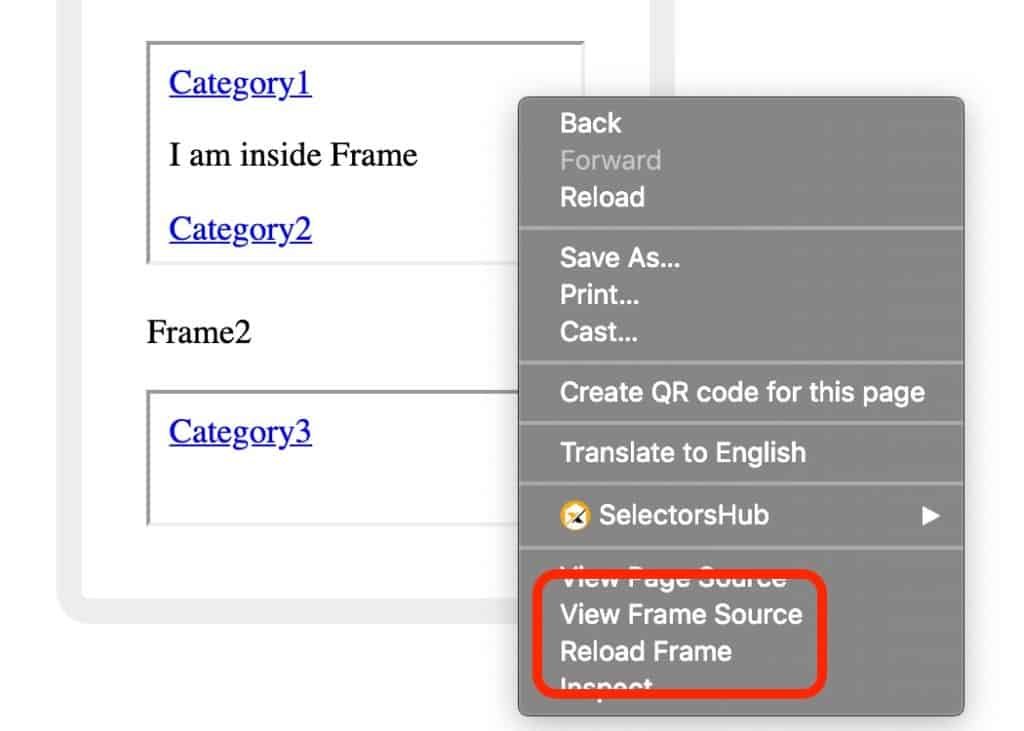
If you get the options like above screenshot, then there are ways we can identify the web elements-
Individual iFrames
driver.switchTo().frame(int x);
//by index, if one or more frames presentdriver.switchTo().frame(String frameName);
//identify the iframe id or name attributedriver.switchTo().frame(WebElement element);
//locate the iframe as webElement and pass the webElement.
Nested iFrames
If there are nested iframes, then we can use
driver.switchTo().frame(ParentFrame).switchTo().frame(ChildFrame);
Out of iFrames
Once complete the action on the iframe elements, if we want to come out of the frame, then dodriver.switchTo().defaultContent();
//to come out of a frame for normal run
Code implementation
We will try to access the text “I am inside frame” text in a normal way (though it’s inside an iframe)
public class Iframes { static WebDriver driver; public static void main(String[] args) throws InterruptedException { System.setProperty("webdriver.chrome.driver", "c:\\Grid\\chromedriver.exe"); driver = new ChromeDriver(); driver.manage().window().maximize(); driver.get("https://skpatro.github.io/demo/iframes/"); String frametext = driver.findElement(By.id("frametext")).getText(); System.out.println(frametext); Thread.sleep(5000); driver.quit(); } }
OutPut
Exception in thread "main" org.openqa.selenium.NoSuchElementException: Unable to locate element:
Now let’s try to access the iframe elements by using driver.switchTo().Frame…
If you inspect the iFrame, we can use id=”Frame1″ or name=”Framename1″
so we can use either of this
driver.switchTo().frame("Frame1");
//frame id
or
driver.switchTo().frame("Framename1");
//frame name
public class Iframes { static WebDriver driver; public static void main(String[] args) throws InterruptedException { System.setProperty("webdriver.chrome.driver", "c:\\Grid\\chromedriver.exe"); driver = new ChromeDriver(); driver.manage().window().maximize(); driver.get("https://skpatro.github.io/demo/iframes/"); driver.switchTo().frame("Frame1"); String frametext = driver.findElement(By.id("frametext")).getText(); System.out.println(frametext); Thread.sleep(5000); driver.switchTo().defaultContent(); driver.findElement(By.linkText("Pavilion")).click(); Thread.sleep(2000); driver.quit(); } }
even we can use,
driver.switchTo().frame("0");
//index wise, switch to 1st iframe
Another way we can achieve switching to iframe by identifying the iframe as webElement –
WebElement firstFrame = driver.findElement(By.id("Frame1")); driver.switchTo().frame(firstFrame); //identify the frame as webelement and pass it as argument
Hope this helps!