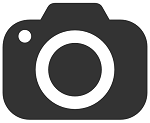
There are scenarios where our test case fails and we use mostly logs to see what actions performed and how our test case failed, but some times when we need to see the screenshot of what exactly happened on the browser while running scripts...
Even manual QA folks take screenshots of the AUT in cases of test case fails or passes… [kind of proof to the execution]
So the same, we can take screenshots in automation as well.
Let’s take the sauceDemo as our AUT.
Consider the valid credentials to login is –
UserName -> qavbox & password -> qavbox1
But as application has a bug and the valid credentials not working, in that case we want to take a screenshot, so we can send the screenshot to dev team for a fix.
After successful login, I should see an element with className -> product_label,
If that element is not present, then catch block will take a screenshot and place it under specified directory.
import java.io.File; import java.io.IOException; import org.openqa.selenium.By; import org.openqa.selenium.OutputType; import org.openqa.selenium.TakesScreenshot; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; import org.apache.commons.io.FileUtils; import java.time.Duration; public class SwagFailureLogin { static WebDriver driver; static WebDriverWait wait; public static void main(String[] args) throws IOException { System.setProperty("webdriver.chrome.driver", "/Users/sunilkumarpatro/sel/chromedriver"); driver = new ChromeDriver(); driver.get("https://www.saucedemo.com/"); driver.manage().deleteAllCookies(); wait = new WebDriverWait(driver, Duration.ofSeconds(5)); driver.findElement(By.id("user-name")).sendKeys("qavbox"); driver.findElement(By.id("password")).sendKeys("qavbox1"); driver.findElement(By.id("login-button")).click(); try{ wait.until(ExpectedConditions.presenceOfElementLocated(By.className("product_label"))); System.out.println("TestCase Pass"); } catch (Exception e) { System.out.println("TestCase Fail" + e.getMessage()); captureScreenshot(); } driver.quit(); } static void captureScreenshot() throws IOException { TakesScreenshot ts = (TakesScreenshot) driver; File f = ts.getScreenshotAs(OutputType.FILE); FileUtils.copyFile(f, new File("./1.jpg")); } }
In the above code block, try block got an exception as the element with className -> product_label not found, catch block called the captureScreenshot() and placed the screenshot under project directory as 1.jpg.
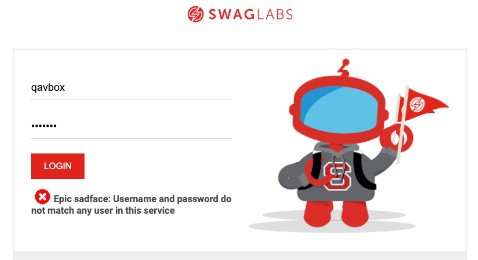
Note – We can use the captureScreenshot() method wherever necessary to take screenshots as well.
Hope this helps, to know more about other screenshot feature, Refer Element or full page screenshot