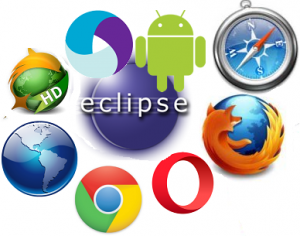
We have seen how to run appium tests on android native apps
In this post, we will be learning how to run appium tests on android handset browsers like Chrome, Firefox, Dolphin or android browser..
application examples on browsers are like facebook.com, gmail.com etc…
for native apps (.apk files), we use uiautomatorviewer.bat to identify elements on the AUT.
In this post, we will be using chrome browser.
NOTE: If chrome browser is not installed on the mobile, then install it from play store,
To install chrome on the emulator such as Genymotion (it might throw error as INSTALL_FAILED_NO_MATCHING_ABIS), follow install ARM translator for setup and install chrome browser using adb command.
Identifying mobile browser elements
Identifying Web elements on the mobile handset browsers and desktop browsers are almost same (follow selenium locators), may be differs the way it appears on mobile browsers…
Open http://qavalidation.com/demo in desktop browser and identify the elements using selenium locators and use the same for appium tests to run on mobile browsers.
we need to change/add certain capabilities for browser automation as compared to tests on native apps,
let’s look into the code implementation
public class Test { static AndroidDriver driver; public static void main(String[] args) throws MalformedURLException, InterruptedException { DesiredCapabilities capabilities = new DesiredCapabilities(); capabilities.setCapability("deviceName", "mygeny510"); capabilities.setCapability(CapabilityType.BROWSER_NAME, "chrome"); capabilities.setCapability(CapabilityType.VERSION, "5.1.0"); capabilities.setCapability("platformName", "android"); driver.get("http://qavalidation.com/demo/"); driver.findElement(By.id("username")).sendKeys("sunil"); Thread.sleep(2000); WebElement Gender = driver.findElement(By.name("sgender")); Select s = new Select(Gender); s.selectByIndex(2); System.out.println("done"); } }
This wouldn’t work. The AndroidDriver is never being instantiated.
//e.g.
AndroidDriver driver = new AndroidDriver(capabilities);
//or
AndroidDriver driver = new AndroidDriver(URL, capabilities);