
There are several situations you might need to open a new tab and load any application to perform certain task or validate something.
We have 2 different ways to deal with this.
Selenium (version >= 4) has this feature out of box for you, you can refer below
Open and handle application in new tab
If you are using selenium version 3, then we can use javascript feature to open a new tab and load the url [applicable for selenium 3 and above]
You can have the url handy or you can grab the href attribute value to get the link in case you want to open any link in new tab of you application.
Javascript uses open() method to create a new tab, syntax
window.open()
This above line we will execute using selenium JavaScriptExecutor, then will load the required url
package locators;import org.openqa.selenium.*;import org.openqa.selenium.chrome.ChromeDriver;import org.openqa.selenium.interactions.Actions;import java.awt.*;import java.awt.event.KeyEvent;import java.util.Set;public class NewTab {public static void main(String[] args) throws InterruptedException, AWTException {System.setProperty("webdriver.chrome.driver", "/Users/myUser/sel/chromedriver");WebDriver driver = new ChromeDriver();driver.get("https://qavbox.github.io/demo/signup/");JavascriptExecutor js = (JavascriptExecutor) driver;js.executeScript("window.open('','_blank');");String parentWindow = driver.getWindowHandle();Set<String> tabs = (Set<String>)driver.getWindowHandles();System.out.println(tabs.size());for(String tab : tabs){if(!tab.equals(parentWindow)) //switch only if it's not parent windowdriver.switchTo().window(tab);driver.get("https://qavbox.github.io/demo/");System.out.println(driver.getTitle());//do some other validation}driver.switchTo().window(parentWindow); //back to parent parentWindow//do somethingThread.sleep(2000);driver.quit();}}
We have opened a new tab.
Then using getWindowHandles() method to capture all the windows, then switching to that tab which is not the parent window [that’s what the for loop does]
If you are sure to navigate to 2nd tab, then you can directly switch to 2nd index tab
driver.switchTo().window(tabs.toArray()[1].toString());//do something on the new tab like loading url, validating somethingdriver.switchTo().window(parentWindow);//do something
Another approach –
You can set the attribute taget = ‘_blank’ for any link of your application using JavaScriptExecutor, which will make the link open in new tab when clicked.
on the demo site – https://qavbox.github.io/demo/signup/3
Let’s add the target attribute of the Home button link so this will open in new tab when clicked.
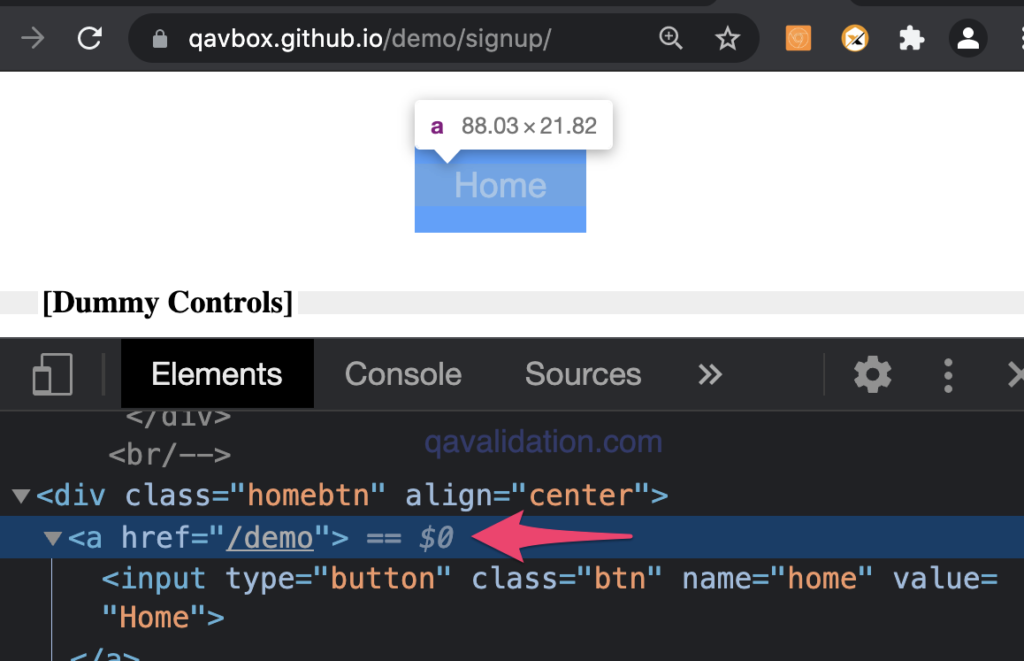
JavascriptExecutor js = (JavascriptExecutor) driver;js.executeScript("document.querySelector('[href=\\'/demo\\']').setAttribute('target', '_blank')");driver.findElement(By.cssSelector("[href='/demo']")).click();Thread.sleep(2000);
Hope this helps!