Browser applications push notifications to user to know their location or push notification when user opens the application on browser.
Why notifications
These notifications are to engage users without knowing their contact details.
Like “know your location” helps to show near by details like weather, restaurants etc near to your area.
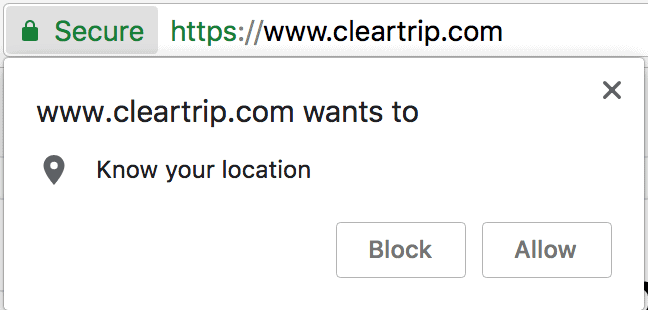
Push notifications help users to get desktop notification when there is a new update.
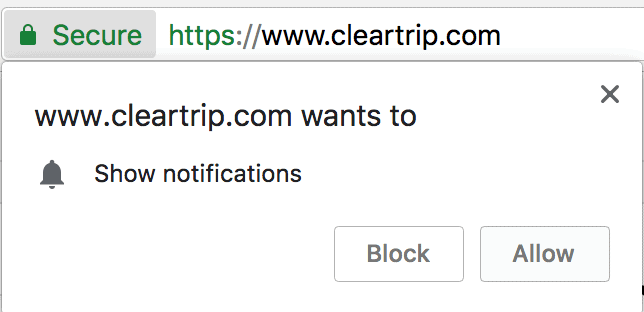
While we are automating the sites that request to allow or block notification, it’s annoying as sometimes you can’t get see the application until you respond to the notification requests.
we will see how we can handle these notifications [allow or block] using selenium
How to handle in selenium
we can use ChromeOptions
for chrome browser & FirefoxOptions
for firefox browser
For chrome browser
To disable “know your location” notification, use
option.addArguments(“disable-geolocation“);
To disable any other browser notifcation, use
option.addArguments(“disable-notifications”);
Let’s see implementation
public class DisableBrowserNotification {public static void main(String[] args) throws InterruptedException {System.setProperty("webdriver.chrome.driver", "/Users/sunilkumarpatro/sel/chromedriver");ChromeOptions option = new ChromeOptions();option.addArguments("disable-geolocation");option.addArguments("disable-notifications");WebDriver driver = new ChromeDriver(option);driver.get("https://cleartrip.com");Thread.sleep(3000);driver.quit();}}
For firefox browser
To disable “know your location” notification, use
option.addPreference(“geo.enabled”, false);
To disable any other browser notifcation, use
option.addPreference(“dom.webnotifications.enabled”, false);
Let’s see implementation
public class DisableBrowserNotification {public static void main(String[] args) throws InterruptedException {System.setProperty("webdriver.gecko.driver", "/Users/myUser/sel/geckodriver");FirefoxOptions option = new FirefoxOptions();option.addPreference("dom.webnotifications.enabled", false);option.addPreference("app.update.enabled", false);option.addPreference("geo.enabled", false);WebDriver driver = new FirefoxDriver(option);//For geo locationdriver.get("https://cleartrip.com");//for show notification//driver.get("https://www.pushwoosh.com/");Thread.sleep(4000);driver.quit();}}
If find helpful, share with friends!
For more topics, refer selenium java tutorial
whoah this blog is wonderful i like studying your articles. Keep up the great work! You recognize, lots of persons are hunting around for this info, you can aid them greatly. |
In the first example, should it be “–disable-notifications”, no? (with the two dashes).
Another question that I have: disabling all the notifications will not affect the upload/download of files?
And: Where do you got the values to pass in the addArguments()/addPreference() methods?
Thanks in advance!