Extent report is one of the flexible API so far, and they have community version as well, so with free of cost you can use with your existing framework.
In this post, we will see how we can do the setup extent reporting and integrate with existing framework. and also will see how to fit into a testNG classes.
Maven dependency
Add following dependency to the pom.xml
<!-- pom.xml --> <dependency> <groupId>com.aventstack</groupId> <artifactId>extentreports</artifactId> <version>3.1.5</version> </dependency>
For basic example and official documentation, refer
Watch the demo here
Folder structure
extentReport
— — TestSample.java
— — ExtentManager.java
— — TestSampleExtent.java
Code samples
TestSample.java
package extentReport; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.Assert; import org.testng.annotations.*; public class TestSample { WebDriver driver; @BeforeTest public void setup() { System.setProperty("webdriver.chrome.driver", "/Users/sunilkumarpatro/sel/chromedriver"); driver = new ChromeDriver(); driver.get("http://qavalidation.com/"); } @Test public void checkSignupPage() { System.out.println("Site Title - " + driver.getTitle()); driver.findElement(By.linkText("DemoForm")).click(); System.out.println("Site Title after clicking link - " + driver.getTitle()); Assert.assertTrue(driver.getTitle().contains("Practice")); } @AfterTest public void tearDown() { driver.quit(); } }
ExtentManager.java
package extentReport; import java.io.IOException; import com.aventstack.extentreports.ExtentReports; import com.aventstack.extentreports.ExtentTest; import com.aventstack.extentreports.MediaEntityBuilder; import com.aventstack.extentreports.Status; import com.aventstack.extentreports.reporter.ExtentHtmlReporter; public class ExtentManager { private static ExtentReports extent; public static ExtentReports createInstance() { // start reporters ExtentHtmlReporter htmlReporter = new ExtentHtmlReporter("extent.html"); //htmlReporter.loadXMLConfig("extent-config.xml"); // create ExtentReports and attach reporter(s) extent = new ExtentReports(); extent.attachReporter(htmlReporter); return extent; } public static ExtentReports getInstance() { if(extent == null) { createInstance(); } return extent; } }
TestSampleExtent.java
package extentReport; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.Assert; import org.testng.annotations.*; import com.aventstack.extentreports.ExtentReports; import com.aventstack.extentreports.ExtentTest; import com.aventstack.extentreports.Status; public class TestSampleExtent { WebDriver driver; private static ExtentTest test; private static ExtentReports extent; @BeforeTest public void setup() { System.setProperty("webdriver.chrome.driver", "/Users/sunilkumarpatro/sel/chromedriver"); driver = new ChromeDriver(); driver.get("http://qavalidation.com/"); extent = ExtentManager.getInstance(); test = extent.createTest(getClass().getName()); } @Test public void checkSignupPage() { //System.out.println("Site Title - " + driver.getTitle()); test.info("Site Title - " + driver.getTitle()); driver.findElement(By.linkText("DemoForm")).click(); test.info("Site Title after clicking link - " + driver.getTitle()); try { Assert.assertTrue(driver.getTitle().contains("Practice1")); } catch(Error e) { //test.fail(e.getMessage()); test.log(Status.FAIL, e.getMessage()); } } @AfterTest public void tearDown() { ExtentManager.getInstance().flush(); driver.quit(); } }
testng.xml
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" > <suite name="Suite1" verbose="1" > <listeners> <listener class-name="extentReport.TestListener"/> </listeners> <test name="testNGListenerTest"> <classes> <class name="extentReport.TestSampleExtentListener" /> </classes> </test> </suite>
Run this testng.xml to run the testcase and generate the html report.
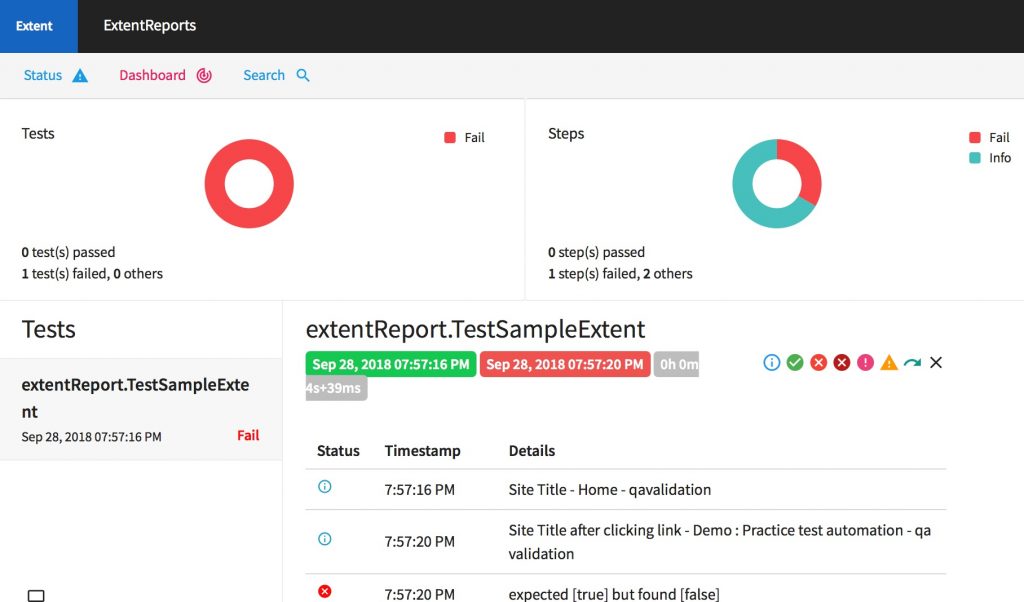
Hope this helps!
Share with friends…