We have already seen many posts on how to run selenium tests in chrome browser by downloading the chromedriver and setting the path.
To run tests in Safari browser, we will use selenium’s SafariDriver class.
Syntax –
WebDriver driver = new SafariDriver(); Driver.get(“https://qavbox.github.io/demo/signup/”)
Note – As per the below link, We no longer needed to download the SafariDriver for safari browsers with version > 10
https://webkit.org/blog/6900/webdriver-support-in-safari-10/
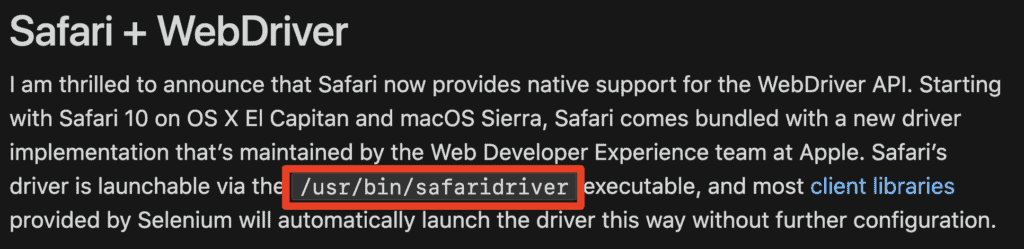
SafariDriver comes default with safari browser, as we upgrade the browser, the driver also upgrades and can be found under
/usr/bin/safaridriver
, so we need not to set the driver in the path.
Here is the complete code implementation –
public class LocatorsSample {public static void main(String []args) throws InterruptedException {WebDriver driver;driver = new SafariDriver();driver.get("https://qavbox.github.io/demo/signup/");driver.findElement(By.cssSelector("input[name='uname']")).sendKeys("QAVBOX");Thread.sleep(2000);driver.quit();}}
You might get below error while running above code –
Exception in thread "main" org.openqa.selenium.SessionNotCreatedException: Could not start a new session. Response code 500. Message: Could not create a session: You must enable the 'Allow Remote Automation' option in Safari's Develop menu to control Safari via WebDriver.
How to fix this above issue –
Display the develop menu bar item –
SafariBrowser > Preferences > Advanced tab > Check the "Show Develop menu in menu bar" box
Navigate for “Allow remote automation”
SafariBrowser > Develop menu item > Select “Allow remote Automation”
If you run the code after above steps done, tests run successfully.
Sometimes you might get error prompt as “This Safari window is remotely controlled by an automated test.”
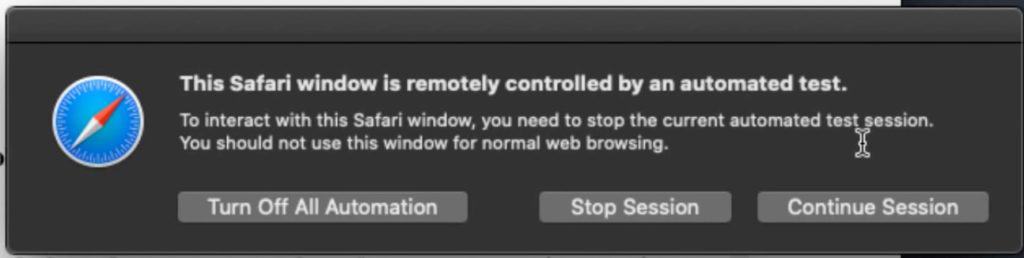
To remove this prompt appear every time, in terminal type and enter
sudo /usr/bin/safaridriver --enable
Note – Enter your system password as prompted
Now you can run selenium tests smoothly.
Hope this helps!