In one of our post, we have discussed why to use docker containers to test or build something.
Selenium has a project called docker-selenium, which provides the out of box docker containers with Java and browser installed, makes it easy to setup and run the tests.
Selenium has 2 different types of docker containers –
- Standalone containers
- Hub & node containers
Standalone containers has both hub and node together in one docker image, so you don’t need to register the node with separate hub image.
but the catch is that only one standalone container can run on a single port at one time, same port can’t be used with more than one standalone containers.
Docker containers download link –
For standalone chrome image – https://hub.docker.com/r/selenium/standalone-chrome
For standalone firefox image – https://hub.docker.com/r/selenium/standalone-firefox
Docker command to pull image and run selenium server –
docker run -d -p 4444:4444 --shm-size="2g" selenium/standalone-chrome:4.7.1-20221208
The above command will download the standalone chrome container and run the selenium the server.
Note – make sure docker desktop should be installed on the system before running above command.
Refer below link to download images for other browsers –
https://github.com/SeleniumHQ/docker-selenium#execution-modes
But the individual docker commands are sometimes tedious when we want to download multiple docker images and sometimes we might not remember the commands.
Docker compose file –
So we will use the docker-compose to write the commands in a .yml file and include with the project, so this can be version controlled with the team members.
Here is an example of docker-compose.yml file to download docker containers with chrome & firefox browser, but the same section can be created for other browsers as well.
Let’s create this file under projectDir/docker directory.
docker-compose-standalone.yml
version: '3.8'services:chrome:image: selenium/standalone-chrome:latesthostname: chromeprivileged: trueshm_size: 2gports:- "4441:4444"- "7900:7900"environment:- SE_VNC_NO_PASSWORD=1- SE_NODE_MAX_SESSIONS=3firefox:image: selenium/standalone-firefox:latesthostname: firefoxprivileged: trueshm_size: 2gports:- "4442:4444"- "7901:7900"environment:- SE_VNC_NO_PASSWORD=1- SE_NODE_MAX_SESSIONS=3
We can use the port as 4444:4444 instead of 4441:4444 / 4442:4444, but we can use only one container with this port.
That’s why we will redirect different port number to hub port – 4441:4444 or 4442:4444
Hub port is always 4444.
“7900:7900”, “7901:7900”
7900 is the port for no vnc browser – to see the live test run on the browser from the docker containers (no need to use vnc utility to see the test run)
SE_VNC_NO_PASSWORD=1
To remove the password when viewing the test run on no vnc browser, else the default password is secret
SE_NODE_MAX_SESSIONS=3
This is run multiple browser instances in parallel, in this case max of 3 browsers can be launched and run the tests in parallel inside the standalone container.
Docker-compose command to download the containers –
In terminal / command prompt, Navigate to ProjectDir/docker and run below command
docker-compose -f docker/docker-compose-standalone.yml up -d
This above command will download the docker containers and run the selenium servers under 4441 port for chrome and 4442 port for firefox.
-d is for detached mode
Dashboard can be viewable under https://localhost:4444
no vnc browser can be viewable under –
http://localhost:7900 for chrome run
http://localhost:7901 for firefox run
We need to update the code as well to fit the selenium server ports under the remoteWebdriver URL.
Refer to this selenium framework github project
We need to update the DriverFactory.java and update as per the ports mentioned under docker-compose file.
if(browser.contains("chrome")){if(App.platform.equalsIgnoreCase("local")){driver = new ChromeDriver();}else if(App.platform.equalsIgnoreCase("remote")){//If you run on dockercap.setBrowserName("chrome");cap.setPlatform(Platform.LINUX);driver = new RemoteWebDriver(new URL("http://localhost:4441/wd/hub"), cap);}}elseif(browser.contains("firefox")){if(App.platform.equalsIgnoreCase("local")){driver = new FirefoxDriver();}else if(App.platform.equalsIgnoreCase("remote")){//If you run on dockercap.setBrowserName("firefox");cap.setPlatform(Platform.LINUX);driver = new RemoteWebDriver(new URL("http://localhost:4442/wd/hub"), cap);//System.out.println("Tests running on " + cap.getBrowserName());}}
Assume selenium java version 4.6 and more, so you don’t need to maintain the browser driver download (SeleniumManager does the job)
To run on chrome, use this url new URL("http://localhost:4441/wd/hub")
To run on firefox, use this url new URL("http://localhost:4442/wd/hub")
Run tests inside containers –
The current framework has different ways to run the tests like
- Using testng.xml
- Using the editor to run individual tests (@Test methods)
- Using maven commands
Above 2 options are meant to run the tests on local browser, but we can run the 3rd option to run on docker which is remote.
Note – you can even the above 2 options as well to run on docker, just that you have to change the default platform value from App.java file.
The above details are explained for the current framework we are discussing, but you can have your own way of implementation.
Run tests with Maven commands –
under terminal > projects directory > run below command
mvn test -Dplatform=remote
you can use any mvn commands to customise your test run like group / package / testng.xml wise.
By default this above command will run on chrome browser.
You can see the test run inside no VNC browser url –
http://localhost:4441/sessions or http://localhost:7900
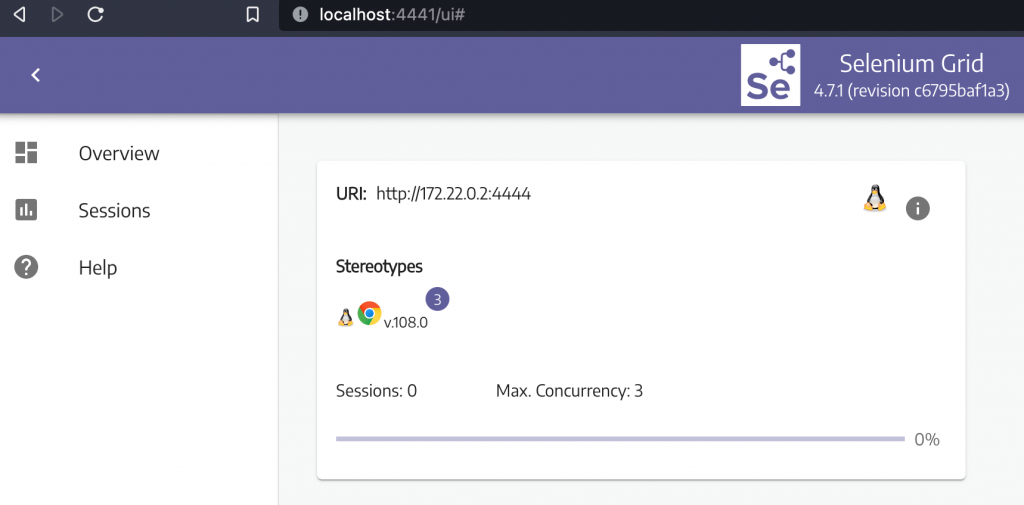
There is no separate Hub here, so we need to navigate to individual grid url to see the dashboard for all browsers individually. like if you want see the selenium grid for firefox browser, you can navigate to url
http//localhost:4442
Click on the Sessions tab to see the test run –
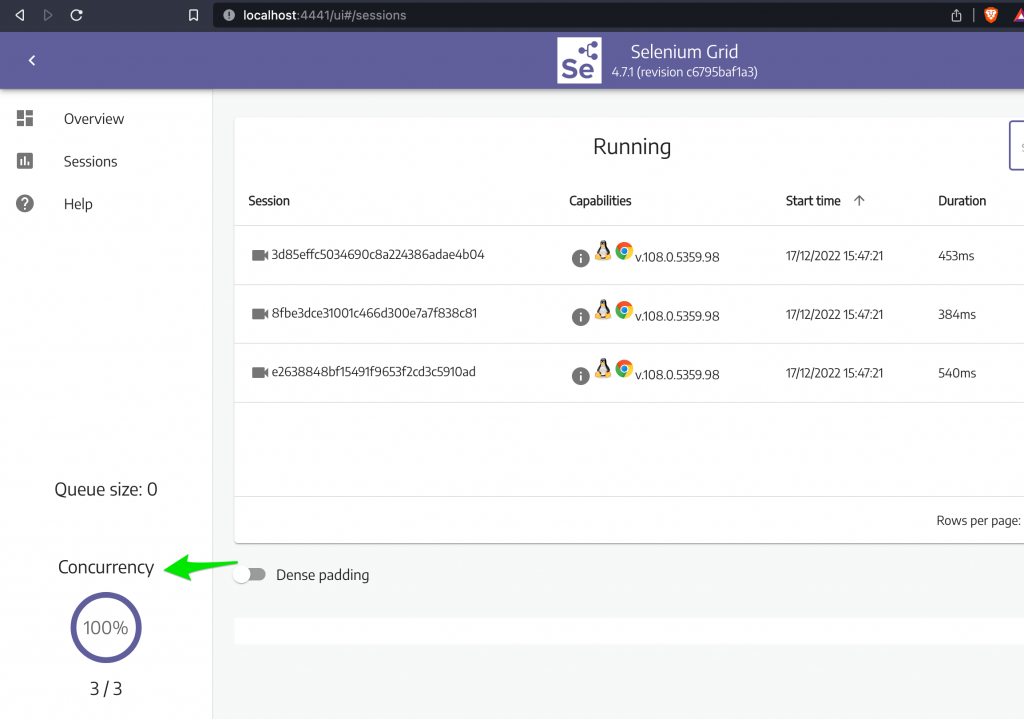
Click on the video icon to see the individual test run.
It won’t ask the password as we have set the SE_VNC_NO_PASSWORD=1, else the password is secret
Stop the docker containers –
docker-compose -f docker/docker-compose-standalone.yml down
Hope this helps!