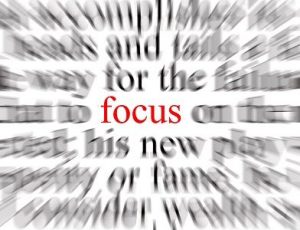
In our one of previous post selenium on textbox, we have seen how to type or send text into textboxes using selenium and java
There are situations where direct sendkeys doesn’t work, so we need to focus on that text box and then sendKeys to type text, this can be achieved by using
- JavaScriptExecutor
- Actions class
Using JavaScriptExecutor
public static void main(String[] args) { System.setProperty("webdriver.chrome.driver","./chromedriver.exe"); WebDriver driver = new ChromeDriver(); driver.manage().window().maximize(); driver.get("http://qavalidation.com/demo/"); JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript("document.getElementById('username').focus();"); }
Using Actions class
public static void main(String[] args) { System.setProperty("webdriver.chrome.driver","./chromedriver.exe"); WebDriver driver = new ChromeDriver(); driver.manage().window().maximize(); driver.get("http://qavalidation.com/demo/"); WebElement uname = driver.findElement(By.id("username")); new Actions(driver).moveToElement(uname).click().perform(); }
Depending on your application, you can use any one of the above methods to focus on text boxes, the same can be done for any of the web elements present on the web browser.
Above methods can be used if in case sendKeys doesn’t work,
sometimes we need to just focus on a textbox and let’s say that sendKeys works perfectly, then you can just use below one line code to focus…
uname.sendKeys(""); //element.sendKeys("")
Very Clear Notes.. Good Example with Js Executor & Actions Class