There are certain scenarios where we want to perform operation on the right click menu options.
let’s see the demo site https://swisnl.github.io/jQuery-contextMenu/demo.html
If you right click on the “right click me” button, it exposes several options to perform, this is what we will automate using selenium.
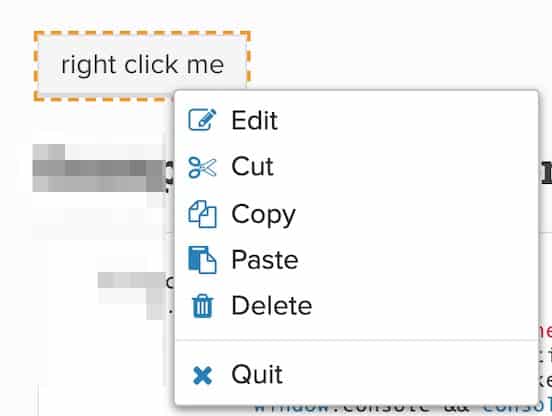
Selenium provides contextClick() which accepts the button to click and then we can use key press events to move up or down for the options and click.
package locators; import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import org.openqa.selenium.support.ui.WebDriverWait; import java.security.Key; import java.time.Duration; public class RightClick { public static void main(String []args) throws InterruptedException { WebDriverWait wait; System.setProperty("webdriver.chrome.driver", "/Users/skpatro/sel/chromedriver"); WebDriver driver; driver = new ChromeDriver(); driver.get("https://swisnl.github.io/jQuery-contextMenu/demo.html"); Thread.sleep(2000); WebElement el = driver.findElement(By.cssSelector(".context-menu-one.btn.btn-neutral")); Actions action = new Actions(driver); action.contextClick(el).sendKeys(Keys.ARROW_DOWN) .pause(Duration.ofMillis(2000)) .sendKeys(Keys.ENTER).build() .perform(); Thread.sleep(2000); System.out.println(driver.switchTo().alert().getText()); driver.quit(); } }
Explanation –
contextClick(webelement el) will pop the menu option, then we will use the Keys.Arrow_down method to move down and keep performing ARROW_DOWN untill we reach to desired option and click using Keys.ENTER method.
above code will click on edit option, and then will pop the alert showing edit confirmation.
Note – We can’t fetch the menu options run times as they expose in HTML DOM, so you need to hard code and write no. of the ARROW_DOWN methods until you reach to desired option.
Hope this helps!
How to right click an element without Actions, Since selenium 3 does not support Actions in FireFox with GeckoDriver