
A basic web table will have these below components:
header, body and data / cells & footer
Header cells- th = Column headers
Body cells – td
Rows – tr
Header and footer are optional for a webtable.
Some of the webpages may or may not have header and footer.
If the theader is present & fetching data by identifying the table element, then be careful to exclude the 1st row while fetching data.
Else, you can directly identify the tbody [or table/tbody] & you will get exact no. of rows that has data [excludes the theader].
Scenario –
Let’s consider this demo site – https://qavbox.github.io/demo/webtable/
To fetch no. rows of a table –
WebElement Table_1 = driver.findElement(By.id("table01")); List Rows = Table_1.findElements(By.tagName("tr")); System.out.println("No. of rows: "+Rows.size());
To fetch the value of each column value or cell in each rows –
for(WebElement Row : Rows) { List Cols = Row.findElements(By.tagName("td")); for(WebElement Cel : Cols) { System.out.print(Cel.getText()+" "); } System.out.println(); }
Scenario –
Check the checkbox in each row where the column value matches “TFS” & click on Delete
We need to get the text of cell data by traverse through each row and column, if the text matches “TFS”, then click on the respective row’s checkbox (index 0 of that row) & then click on the Delete button.
for(int i=0; i< rows.size() ; i++) { List cols = rows.get(i).findElements(By.tagName("td")); for(int j=0; j < cols.size() ; j++) { if(cols.get(j).getText().equals("TFS")) { cols.get(0).findElement(By.tagName("input")).click(); //checkbox is present on 0th index cols.get(4).findElement(By.tagName("input")).click(); //Delete button is present on 4th index } } }
Complete code implementation –
package controls;import java.util.List;import org.openqa.selenium.By;import org.openqa.selenium.WebDriver;import org.openqa.selenium.WebElement;import org.openqa.selenium.firefox.FirefoxDriver;public class Table1 {public static void main(String[] args) {WebDriver driver = new ChromeDriver();driver.get("https://qavbox.github.io/demo/webtable/");driver.manage().window().maximize();WebElement Table_1 = driver.findElement(By.id("table01"));List<webelement> Rows = Table_1.findElements(By.tagName("tr"));System.out.println("No. of rows: "+Rows.size());//to print all the values inside the table/*for(WebElement Row : Rows){List<webelement> Cols = Row.findElements(By.tagName("td"));for(WebElement Cel : Cols){System.out.print(Cel.getText()+" ");}System.out.println();}*///Find a matching text in a table and check the checkbox and click on delete button//using the mainloop: as I know there is only row data whose value is "TFS", then I//stop the loop i.e- break mainloop//If you know there are multiple rows matching with "TFS", then remove the mainloopmainloop:for(int i=0; i<Rows.size();i++){List<webelement> Cols = Rows.get(i).findElements(By.tagName("td"));for(int j=0; j<Cols.size();j++){if(Cols.get(j).getText().contains("TFS")){Cols.get(0).findElement(By.tagName("input")).click();Cols.get(4).findElement(By.tagName("input")).click();break mainloop;}}}}}
Another way of fetching table data – based on TR TD index –
use of xpath: if we inspect webtable cells with any inspection tools like selectorshub on webpage, the xpath mostly remains same except the index of tr and td tag…
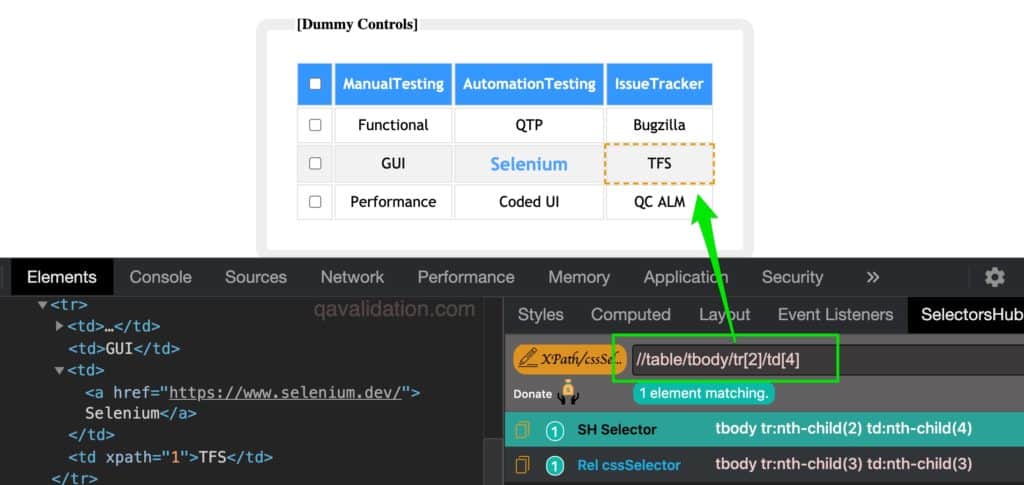
As you can see in above screenshot, item TFS is on 2nd row and 4th column…
so we can use 2 for loops to iterate the tr and td tags to get the cell values based on the row & column index
let’s see it in action –
String cell=null;for(int i=0;i<Rows.size();i++){List cols=Rows.get(i).findElements(By.tagName("td"));for(int j=0;j<cols.size();j++){cell = driver.findElement(By.xpath(".//*[@id='table01']/tbody/tr["+i+"]/td["+j+"]")).getText();System.out.print(cell);}System.out.println();}
Hope this helps!