In this post, we will see how to handle / automate drop down webelement in Selenium and Java.
Use cases would be –
- select an option from the drop down
- Fetch all the options.
- Fetch the selected option
A dropdown element on browser looks like below –
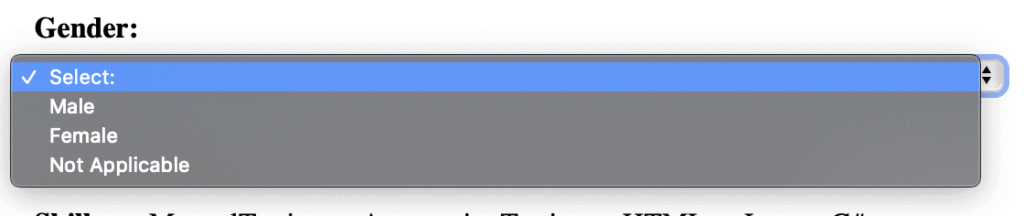
The HTML associated with select or dropdown is –
<select name="sgender"> <option value="select">Select:</option> <option value="male">Male</option> <option value="female">Female</option> <option value="na">Not Applicable</option> </select>
Dropdown elements are noted as select tag
in html, each select tag has items noted as options tag
Selenium provides 3 different ways to select an option from dropdown –
select.selectByIndex(int index); select.selectByValue("valueAttribute"); select.selectByVisibleText("visibletext");
To fetch the selected option [WebElement], we use
select.getFirstSelectedOption()
To get the selected option text, we can use
select.getFirstSelectedOption().getAttribute("value") or select.getFirstSelectedOption().gettext()
To fetch all the items from the dropdown, we use
select.getOptions()
We will use the Gender field of this demo site – https://qavbox.github.io/demo/signup/
To select Not Applicable option from the dropdown, we can use index or value attribute or option text
Also display the selected option text from dropdown
package elements; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.List; public class DropDownSample { public static void main(String []args) throws InterruptedException { System.setProperty("webdriver.chrome.driver", "/Users/UserName/sel/chromedriver"); WebDriver driver; driver = new ChromeDriver(); //launch browser driver.get("https://qavbox.github.io/demo/signup/"); //loads url Thread.sleep(3000); WebElement dropdowEl = driver.findElement(By.name("sgender")); Select select = new Select(dropdowEl); select.selectByIndex(3); //or // select.selectByValue("na"); //or // select.selectByVisibleText("Not Applicable"); System.out.println(select.getFirstSelectedOption().getText()); //or System.out.println(select.getFirstSelectedOption().getAttribute("value")); Thread.sleep(2000); driver.quit(); } }
Identify the dropdown element and then pass it to Select class constructor
WebElement dropdowEl = driver.findElement(By.name("sgender"));
Select select = new Select(dropdowEl);
let’s see the HTML or Not Applicable option –
<option value="na">Not Applicable</option>
Here the value is “na”
Display text is – Note Applicable
Index is – 3 [starts from 0]
To fetch all available options from the dropdown, no. of options and display the text
List<WebElement> options = select.getOptions(); System.out.println(options.size()); for(WebElement el : options){ System.out.println(el.getText()); //if you want to select an option whose text is "Male" if(el.getText().equals("Male")){ el.click(); break; } }
Note with dropdown, you can select only one value at a time, if you have a list box then you can select multiple options,
for this refer automate list box using selenium java
Hope this helps!
Hi can u show the how to click the invisible element from boot strap drop down
If an option is invisible, you can’t select, you have to scroll down till the element is visible and then select
refer and try any one ways from http://qavalidation.com/2015/09/play-with-scroll-bars-on-a-page-in-selenium-and-javascript.html/