In this post, we will see how to handle drop down webelement in Selenium and python, select an option from the drop down & also fetch all the options.
Prerequisite
Configure & run python on PyCharm [my preference]
Know how to write Selenium test using Python
How to select an item
A dropdown DOM look like this, the html tag is select, which will have option as child elements that represent the dropdown items
<select name="sgender"><option value="select">Select:</option><option value="male">Male</option><option value="female">Female</option><option value="na">Not Applicable</option></select>
First identify the select
element
then use options
method to get list of options available and select an option by using available methods as
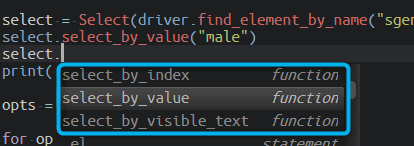
We have 3 ways to select an option –
select_by_index
we can select an option based on the index, starting from 0
Below code line will select the 1st option from the dropdown –
selectElement.select_by_index(0)
select_by_value
This method will select the option whose value
attribute matches to the string provided
<select name="sgender">
<option value="select">Select:</option>
<option value="male
">Male</option>
selectElement.select_by_value("male")
select_by_visible_text
This above method will select the option whose visible text matches to the string provided
To select Female from the drop down
selectElement.select_by_visible_text("Female")
Fetch the selected item
Python library provides a property first_selected_option
that returns the element or item selected from the dropdown
print("\nSelected option - " + select.first_selected_option.text)
.text will print the item text.
To fetch all the dropdown items
Python library provides property as options
which will return all the drop down items as elements
select.options
We can loop to get individual items and their text.
opts = select.optionsprint("Available options are - ")for opt in opts:print(opt.text)
opt.text will print the text present form the option tag.
Let’s see code implementation in action
In selenium with python, we need to import the Select class
from selenium.webdriver.support.ui import Selectfrom selenium import webdriverfrom selenium.webdriver.common.by import Byfrom selenium.webdriver.support.ui import Selectimport timedriver = webdriver.Chrome("C:\\Grid\\chromedriver.exe")driver.maximize_window()driver.get("https://qavbox.github.io/demo")driver.find_element_by_link_text("SignUp Form").click()select = Select(driver.find_element_by_name("sgender"))select.select_by_value("male")print("Selected option " + select.first_selected_option.text)opts = select.optionsprint("Available options are")for opt in opts:print(opt.text)time.sleep(3)driver.quit()
If you want to select an option directly without using the Select class, you can achieve it by using xpath [get the tag ‘select’ and then it’s child tag ‘option’], as
driver.find_element_by_xpath("//select[@name='sgender']/option[@value='female']").click()