In our previous post, we have seen the features / enhancements to selenium 4.
Selenium 4 provides taking
- Screenshot for full page [available for FireFoxDriver]
- screenshot for a particular element[s]
- If you are taking screenshot for a parent element, then all the child elements also appear on the screenshot
Before to selenium 4, we use to use some other java library to achieve the element screenshot or full page screenshot, like using Ashot library
Now selenium 4 supports taking element[s] screenshot out of box & full page screenshot for firefox driver.
Note – You can use Ashot library for taking full page screenshot [other than firefox browser]
Element[s] screenshot
Now with selenium 4, WebElement has a new method getScreenshotAs()
to take screenshot.
Element screenshot will help to take only the elements which are having issue on screen or you want to take screenshot only the portion of screen to show to dev team or to log a defect, if you are taking a page screenshot, then you have to crop the required portion and attach is defect which might take some time and some times the dev or analyst may not be sure which element on the screen has the issue if you are providing a full page screenshot.
To demo this, we will be taking saucedemo website
Let’s consider a scenario, if the login is unsuccessful, we will be taking screenshot of error message along with the cross icon,
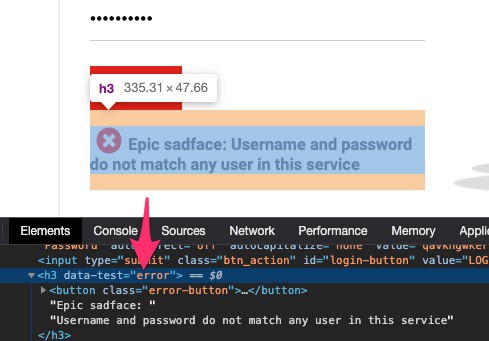
To verify if the login successful, we will be checking if the browser shows the screen with “Products” text
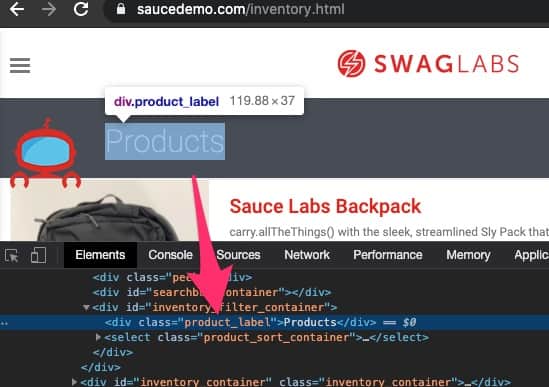
We will be crating utility method captureScreenShotOf(WebElement el, WebDriver driver), pass the element for which you want to take the screenshot, you can keep this method where all your common methods are present and call where ever you need
void captureScreenShotOf(WebElement el, WebDriver driver){ File newImg = el.getScreenshotAs(OutputType.FILE); try{ FileUtils.copyFile(newImg, new File("./screenshot/ElPageShot.jpg")); }catch(Exception e){ e.printStackTrace(); } }
In this post, we will be calling to our test
package sel4; import io.github.bonigarcia.wdm.WebDriverManager; import org.apache.commons.io.FileUtils; import org.openqa.selenium.*; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; import org.testng.annotations.BeforeMethod; import org.testng.annotations.Test; import javax.imageio.ImageIO; import java.io.File; import java.io.IOException; import java.time.Duration; public class ScreenshotSamples { private static WebDriver driver; private static WebDriverWait wait; @BeforeMethod void Login() throws InterruptedException { //WebDriverManager.chromedriver().setup(); //driver = new ChromeDriver(); WebDriverManager.firefoxdriver().setup(); driver = new FirefoxDriver(); driver.get("https://www.saucedemo.com/"); Thread.sleep(2000); wait = new WebDriverWait(driver, Duration.ofSeconds(5)); } @Test public void ElScreenshot() throws InterruptedException { driver.manage().window().minimize(); driver.findElement(By.id("user-name")).sendKeys("qavbox"); driver.findElement(By.id("password")).sendKeys("qavbox1"); driver.findElement(By.id("login-button")).click(); try { wait.until(ExpectedConditions.presenceOfElementLocated(By.className("product_label"))); } catch (Exception e) { System.out.println("Login Error" + e.getMessage()); WebElement el = driver.findElement(By.cssSelector("[data-test='error']")); captureScreenShotOf(el, driver); } Thread.sleep(2000); driver.quit(); } }
In above code, if the Product text not found, it means that our login failed and we will be taking the screenshot of the displayed error portion of screen.
Note – You can use the screenshot method as it fits to your need.
Full page screenshot
With Selenium 4, FirefoxDriver includes a new method getFullPageScreenshotAs()
to take full page screenshot
Here is the utility method –
void captureFullPageShot(WebDriver driver){ File newImg = ((FirefoxDriver) driver).getFullPageScreenshotAs(OutputType.FILE); try { FileUtils.copyFile(newImg, new File("./screenshot/FullPageShot.jpg")); } catch (Exception e) { e.printStackTrace(); } }
This method accepts the driver, and then we are casting to FirefoxDriver.
You can call this method whenever you want to take a full page screenshot, even you can customise further by adding another parameter to this method as
getFullPageScreenshotAs(WebDriver driver, String filePath)
Now lets say we want to take full page screenshot for browsers other than Firefox, then we can use Ashot library
You need to add the maven dependency into your pom.xml, you can get from here
This below utility method you can use to take full page screenshot irrespective of browser
static void captureFullPageShot_AnyBrowser(WebDriver driver){ Screenshot screenshot = new AShot().shootingStrategy(ShootingStrategies.viewportPasting(1000)).takeScreenshot(driver); try { ImageIO.write(screenshot.getImage(), "PNG", new File("./screenshot/FullPageShot_Any.png")); } catch (IOException e) { e.printStackTrace(); } }
1 Comment