In selenium, we all know findElement() method finds the elements based on the locator matched criteria from the browser application.
There are 2 ways we can use the findElement(), with WebDriver and also with WebElement
In this post, we will see the syntax and outcome of each ways
For this explanation, I will be using qavbox demo
We will use findelement() with driver object and see how many ‘li’ elements are present and then we will use the findelement() with webelement id=subgroup to see what different result we get.
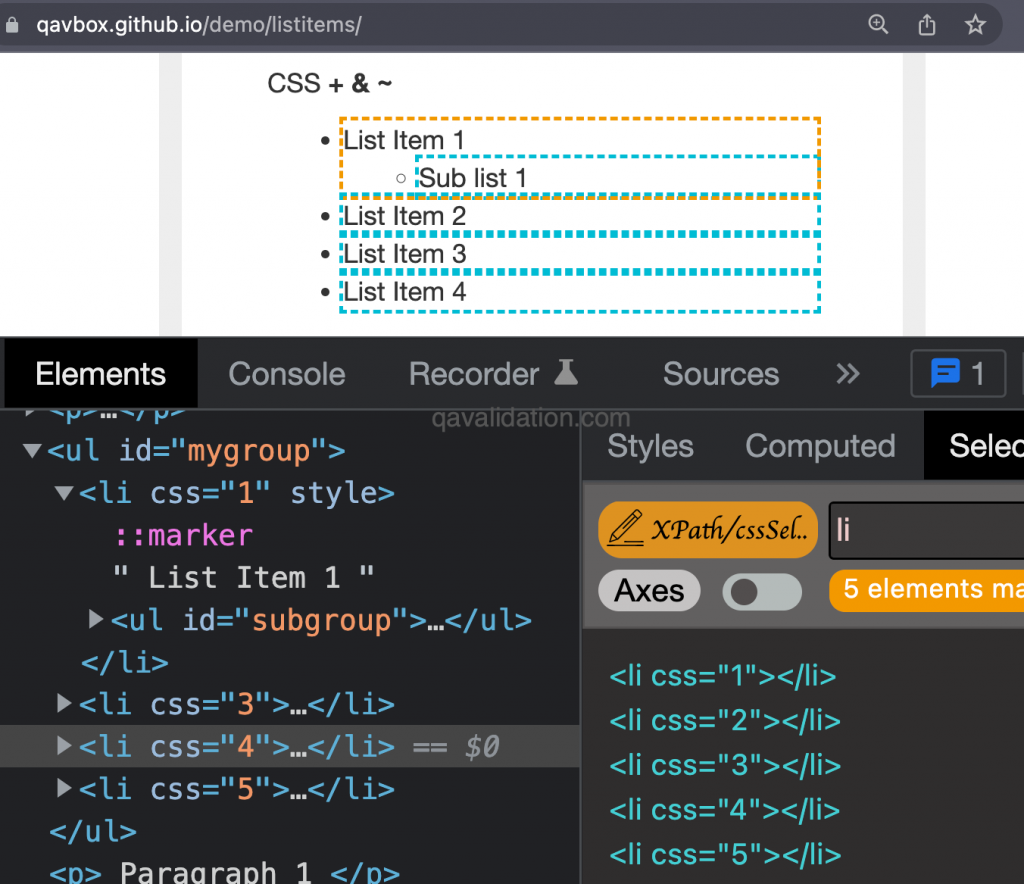
driver.findElement()
A sample code block explaining the driver.findElement()
driver.get("https://qavbox.github.io/demo/listitems/");List<WebElement> items =driver.findElements(By.tagName("li"));System.out.println(items.size());
Output – 5
driver.findElements(By.tagName(“li”)) basically searches for all the li elements present on the current webpage / screen not on entire application or other screens.
With an exception, driver excludes the elements present inside iFrame and shadowRoot. (we need to handle these elements in a different way)
WebElement.findElement()
A sample code block explaining the element.findElement()
driver.get("https://qavbox.github.io/demo/listitems/");WebElement element =driver.findElement(By.id("subgroup"));List<WebElement> items =element.findElements(By.tagName("li"));System.out.println(items.size());
Output – 1
element.findElements() searches for any child elements with tagname “li” under the ‘element’ (with id ‘subgroup’) not on entire screen.