In one of our previous post, we have seen how to use default testNG html report to understand the pass, failure and skip tests and debug the tests.
In this post we will be seeing how to send log messages and attach screenshots to emailable-report.html and index.html
We will use Reporter api to achieve this.
Reporter class has one overloaded method log() to send messages to console output and also to html report.
log(java.lang.String s, boolean logToStandardOut)
logToStandardOut should be true, if you want to send messages to console output and also to html report.
You can use Reporter.log() method inside testNG methods directly, but if you want to use this method testng annotations like @AfterMethod, then you need to use below statement before using Reporter.log
@AfterMethod public void failureSetup(ITestResult result) throws IOException { Reporter.setCurrentTestResult(result); //to-do }
With ITestListener implemented methods, you can directly use Reporter.log()
Let’s look into the implementation of Reporter.log() to send messages and attach screenshot –
BaseTest.java
package testNgLearning.reporterLog; import org.openqa.selenium.OutputType; import org.openqa.selenium.TakesScreenshot; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.ITestResult; import org.testng.Reporter; import org.testng.annotations.AfterMethod; import org.testng.annotations.BeforeClass; import java.io.File; import java.io.FileOutputStream; import java.io.IOException; public class Basetest { WebDriver driver; @BeforeClass public void setup(){ //Reporter.setCurrentTestResult(result); System.setProperty("webdriver.chrome.driver","/Users/skpatro/sel/chromedriver"); driver = new ChromeDriver(); Reporter.log("empty browser launched"); } @AfterMethod public void failureSetup(ITestResult result) throws IOException { Reporter.setCurrentTestResult(result); File img = new File(System.getProperty("user.dir")+"/screen_"+result.getMethod().getMethodName()+".png"); if(result.getStatus() == 2){ //failed scenaario Reporter.log("This is failed log from reporter.log", true); FileOutputStream screenshotStream = new FileOutputStream(img); screenshotStream.write(((TakesScreenshot) driver).getScreenshotAs(OutputType.BYTES)); screenshotStream.close(); Reporter.log(" <a href='"+img.getAbsolutePath()+"'> <img src='"+ img.getAbsolutePath()+"' height='200' width='200'/> </a> "); } } }
BaseTest > @BeforeClass method – Driver is initialised, so other testNG classes can use it.
@AfterMethod – logic written to check if the current test failed, then take the screenshot
if(result.getStatus() == 2){....}
for failed test
result.getStatus() will return
- 1 – for passed test
- 2 – for failed test
- 3 – for skip test
- 4 – SUCCESS_PERCENTAGE_FAILURE
- 16 – test started
Using Reporter.log(" <a href-'"+img.getAbsolutePath()...
we are sending html content (link with image path) as string to html report.
SeleniumTest.java
package testNgLearning.reporterLog; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.Assert; import org.testng.Reporter; import org.testng.annotations.Listeners; import org.testng.annotations.Test; //@Listeners(TestNGListenerManager.class) //public class SeleniumTest{ public class SeleniumTest extends Basetest{ //Pass scenario @Test public void MavenParamTest() throws InterruptedException { driver.get("https://qavbox.github.io/demo/links/"); Reporter.log(driver.getTitle(), true); Thread.sleep(2000); } //Fail scenario @Test public void MavenParamTest_fail() throws InterruptedException { driver.get("https://qavbox.github.io/demo"); Reporter.log(driver.getTitle(), true); Assert.fail(); Thread.sleep(2000); } }
This above testNG class is extending the BaseTest class, so the driver instances are shared to each test method.
and for each test run, AfterMethod will be run, if failed, then it will attach screenshot to html report.
Run the SeleniumTest.java
After running, navigate to project root folder > test-output folder > open the emailable-report.html and index.html
You will see the logs and attached screenshot
emailable-report.html –
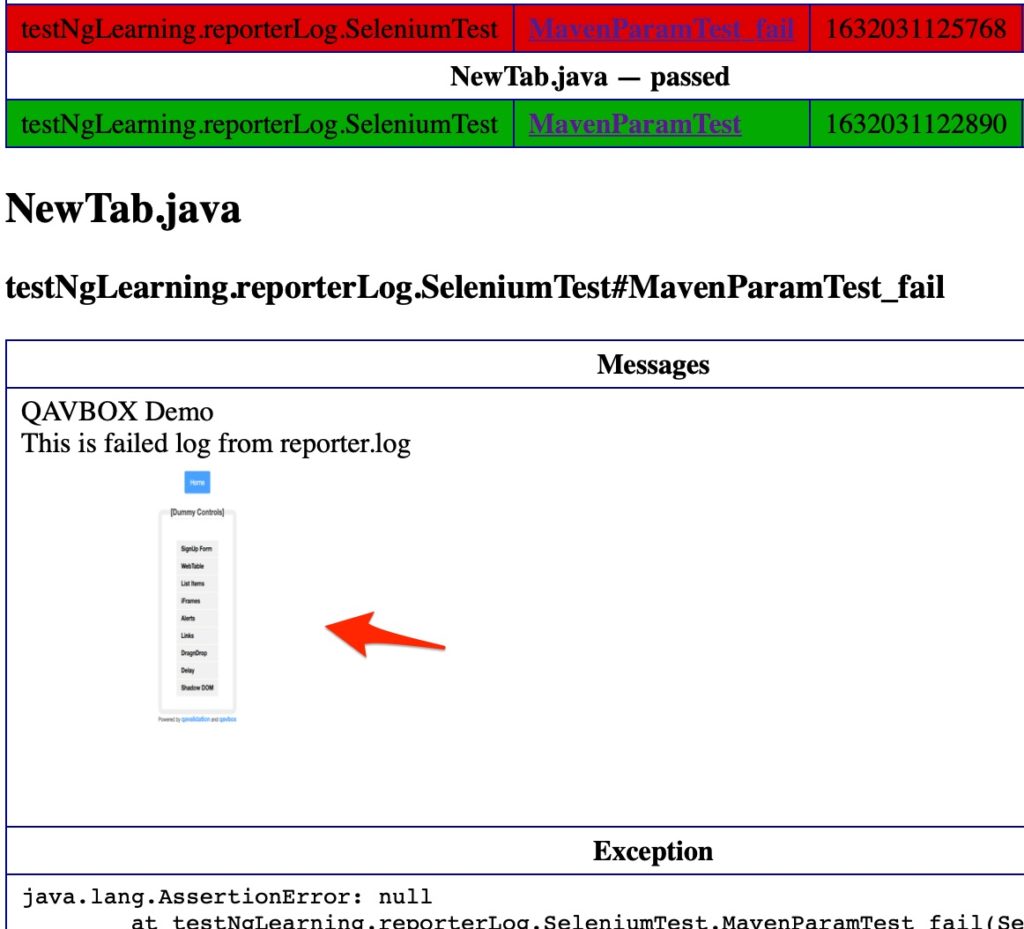
index.html
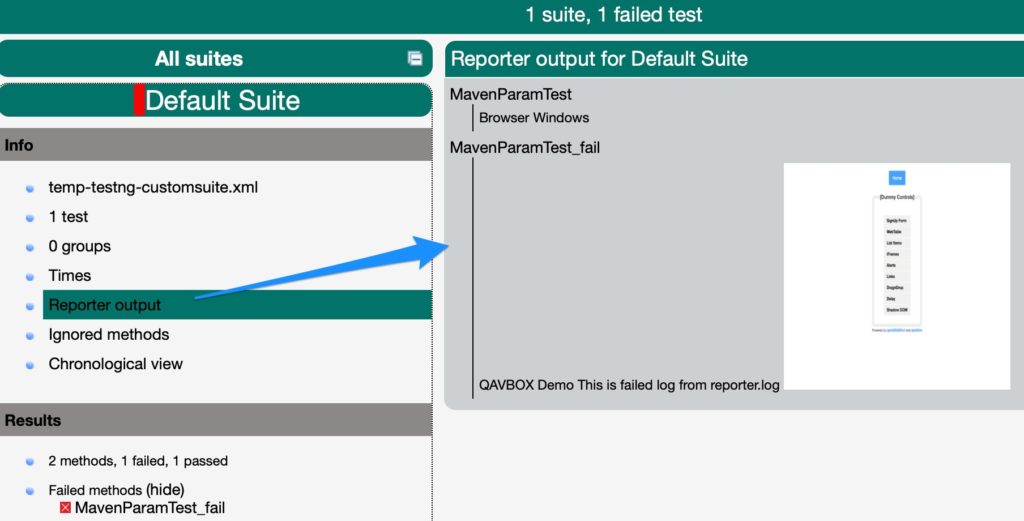
The same way, you can even use the Reporter.log method in ITestListener
implemented methods
Write the driver initialisation code in onTestStart()
method And in the onTestFailure()
method write the logic of taking screenshot and Reporter.log method to attach screenshot
Find the code implementation here, watch above video for detailed explanation.
Hope this helps!